The Requirements: To clear the contents of a range.
The Data: The data involved contains a mix of cells (i.e:, some cells have content, others are empty), as could be understood from this line in the question code While Cells (Num_Ligne, 3) <>""
.
The figure below represents a sample of the data. There we have cells with a constant value (X), cells with formulas (filled with a pattern), and all other cells are empty. In this sample data the Last Row is the Row 26.
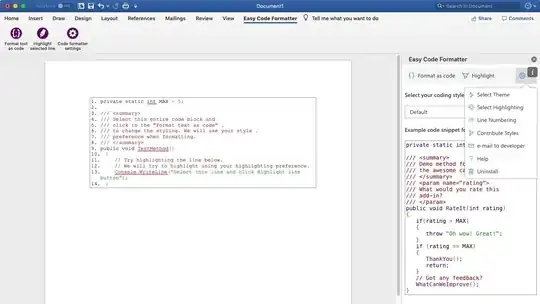
Now let's look at some of the methods to obtain the last row in line with the data involved.
End(xlUp).Row method: In this case, I would suggest not using this method unless it is applied to each of the columns involved, but then it won't be practical.
When applied only to the first column, it returns row 25, instead of row 26.
Find method: In this case, this method will only be effective if it is applied to the entire range.
When applied only to the first column, it also returns row 25.
When applied to the entire range [C:J]
it will return the correct row 26.
However, if the last time the Find
method was utilized the SearchOrder
applied was xlByColumns
, then when we run our procedure the method will return row 25, if the SearchOrder
was not set to xlByRows
in our procedure.
Bear in mind that the LookIn
, LookAt
, SearchOrder
and MatchByte
* parameters are saved each time the Find
method is applied, therefore these parameters should always be included (*if applicable) to ensure the expected return.
UsedRange: This is one of the few cases where this worksheet property could be used. As in this case, the objective is to set a range from an initial row to the last row with contents of specific columns and then clear the contents of that range. Actually utilizing the UsedRange
simplifies the code a lot.
With ActiveSheet
Range(.Rows(8), .Rows(.UsedRange.SpecialCells(xlCellTypeLastCell).Row)).Columns("C:J").ClearContents
End With
Last Row? However, each problem has its own peculiarities, and for this particular problem we should also ask ourselves the question:
- In this case, is the last row really necessary?
I think the answer is no. So let's simplify things even more.
With ActiveSheet
Range(.Rows(8), .Rows(.Rows.Count)).Columns("C:J").ClearContents
End With