you're depending on the browser to process that HTML attribute, make sure that is a supported feature of all browsers you want your app/site to be visible
regarding Cypress, there's not much it can not do and remember that in Cypress/Puppeteer/etc you can pass a path to the element, like a[download]
here's the running test
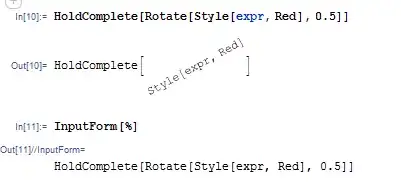
Cypress can easily test downloadable files since version 6.3.0
./index.html
<!DOCTYPE html>
<html>
<body>
<h1>The a download attribute</h1>
<p>Click on the image to download it:<p>
<a href="/myw3schoolsimage.jpg" download>
<img src="/myw3schoolsimage.jpg" alt="W3Schools" width="104" height="142">
</a>
<p><b>Note:</b> The download attribute is not supported in IE or Edge (prior version 18), or in Safari (prior version 10.1).</p>
</body>
</html>
./cypress/plugins/index.js
module.exports = (on, config) => {
on('task', {
deleteFolder (folderName) {
console.log('deleting folder %s', folderName)
return new Promise((resolve, reject) => {
rmdir(folderName, { maxRetries: 10, recursive: true }, (err) => {
if (err) {
console.error(err)
return reject(err)
}
resolve(null)
})
})
},
})
}
./cypress/integration/w3schools.specs.js
const path = require('path')
const deleteDownloadsFolder = () => {
const downloadsFolder = Cypress.config('downloadsFolder')
cy.task('deleteFolder', downloadsFolder)
}
describe('My First Test', () => {
const downloadsFolder = Cypress.config('downloadsFolder')
beforeEach(deleteDownloadsFolder)
it('Visit w3schools example', () => {
cy.visit('/index.html')
cy.get('a[download]').click()
cy.log('**read downloaded file**')
const filename = path.join(downloadsFolder, 'myw3schoolsimage.jpg')
cy.readFile(filename, { timeout: 15000 })
.should('have.length.gt', 50)
})
})