Testing your localhost app from anther device
ngrok
is a tunneling tool that creates public URLs that allow access to your application from another computer. Typically, your computer has a firewall which prevents access to your application.
To use it, you will need to install it:
(venv)$ pip3 install pyngrok
pyngrok
is a Python wrapper for ngrok
that manages its own binary and puts it on your path, making ngrok
readily available from anywhere on the command line and via a convenient Python API.
Run ngrok --help
in your virtual environment to confirm that it is installed and working:
(venv)$ ngrok --help
How it works
Open two terminal windows:
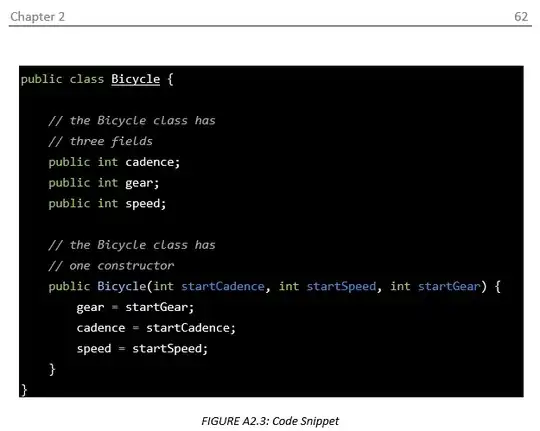
If you do not know how to do this on your default ubuntu terminal window, consider using the byobu window manager and terminal multiplexer. Alternatively, if you are on VSCode, you can simply split your terminal.
- On your first window, start your application to access
http://127.0.0.1:5000/
- On your second terminal, start
ngrok
:
(venv)$ ngrok http 5000
# Or any other port you may be using
From the second window running ngrok
, look for lines beginning with "Forwarding". ngrok
provides you with both http
and https
versions of free publicly accessible URLs. You might have something such as http://23ebfb1baa7e.ngrok.io
.
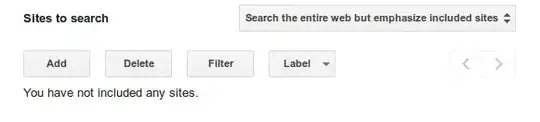
The subdomain portion is going to be different each time you run ngrok
. Send this URL to anther device to access your locally running application. It works from anywhere in the world!
This is the quickest way you can access your application while it is still in development.
Going further: How to create a ngrok tunnel
It is exciting to know that you can actually run your application on another device. The method that I have shown you above will require you to do this every time you want to access your application. But you might want to automatically create ngrok
tunnel every time you fire up your server. I will show you an example using the flask server.
In your application instance (app/init.py), use ngrok.connect
to return a free public URL that is assigned to your tunnel.
def start_ngrok():
from pyngrok import ngrok
url = ngrok.connect(5000).public_url
print('* Tunnel: ', url)
if app.config['START_NGROK']:
start_ngrok()
As soon as we have defined the function which handles the ngrok
tunnel, we check if its configuration has been set in our environment variables. In your config.py
file, create an instance of START_NGROK
:
START_NGROK = os.environ.get('START_NGROK') is not None
Then in a hidden file that has all your environment variables (we can call it .flaskenv
), define your variable:
# .flaskenv
START_NGROK=1
If you use a reloader and have enabled the ngrok
tunnel, you will notice that two URLs are provided as soon as you fire up your server.
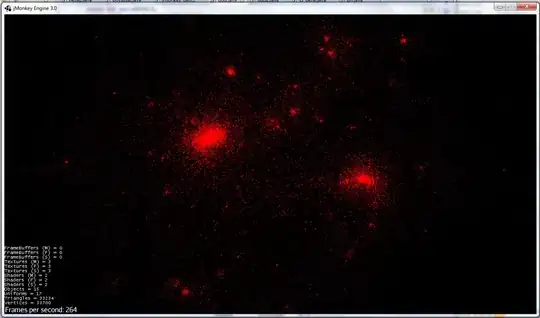
Use the URLs on another device to access your locally running application.
For clarity, this is the kind of application structure I am referring to when building a flask application:
project_folder
| ---------- config.py
| ---------- app.py
| ---------- .flaskenv
| ---------- app/
| ---------- routes.py
| ---------- __init__.py
| ---------- models.py
| ---------- templates/
| ---------- static/