You can accomplish all of this with .loc. First, loop through all of the unique item numbers and use .loc to transform a subset of columns. Then use .loc to select only rows where Total < PV Pick.
import pandas as pd
df = pd.DataFrame({'Item Number': ['104430-003', '104430-003', '104430-003', 'VTHY-039', 'VTHY-039', 'VTHY-039', 'VTHY-039'],
'PV Pick': [4, 4, 4, 1, 1, 1, 1],
'Items': [2, 4, 1, 2, 2, 2, 3]})
items = df['Item Number'].unique()
for item in items:
df.loc[df['Item Number'] == item, 'Total'] = df.loc[df['Item Number'] == item, 'Items'].cumsum()
df = df.loc[df['Total'] < df['PV Pick']]
This is what the output looks like after running the for loop:
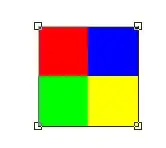
And after selecting rows where Total < PV Pick:

EDIT:
To answer your comment, in the loop you can use .loc to find rows where 'Total' >= 'PV Pick', grab just the first row with iloc, and append these values to breakeven_final. Then you can concat df with df_breakeven_final.
breakeven_final = []
for item in items:
df.loc[df['Item Number'] == item, 'Total'] = df.loc[df['Item Number'] == item, 'Items'].cumsum()
breakeven = df.loc[df['Item Number'] == item].loc[df['Total'] >= df['PV Pick']].iloc[0]
breakeven_final.append(breakeven.values)
df_breakeven_final = pd.DataFrame(breakeven_final, columns=df.columns.values)
df = df.loc[df['Total'] < df['PV Pick']]
df_final = pd.concat([df, df_breakeven_final]).reset_index(drop=True)
Output of df_final (note the index has been reset):
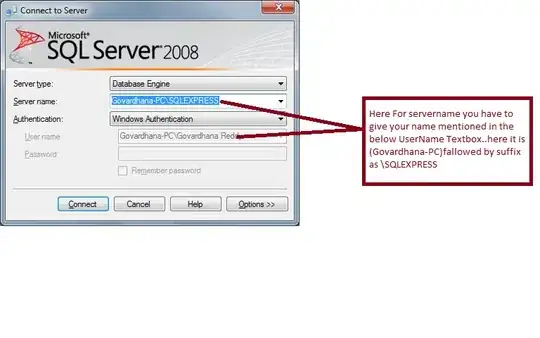