If you are looking for a way to create add-ons in Apps Script, you might want to check this post
In order to use the add-on in other files, you would have to do one of the following:
(1) Publish the add-on, as explained here.
(2) Test the add-on via Run > Test as add-on...
. I wouldn't recommend this, since you would have to add each file you want to use the add-on with first, and open the file from there.
It was mentioned that to use the add-on to different files, you need to publish your add-on which needs to pass the add-on publication requirements
One workaround I could suggest is to copy your apps script code to specific document file programmatically using Apps Script API. You can choose your preferred programming language to use this API, one example would be Python Quickstart
What you need to do?
- create an apps script project using projects.create. You just need to provide the script
title
and the parentId
in the request body
Note:
parentId : The Drive ID of a parent file that the created script project is bound to. This is usually the ID of a Google Doc, Google Sheet, Google Form, or Google Slides file. If not set, a standalone script project is created.
Sample Request Body:
{
"title": "InboundScript",
"parentId": "1_XlY8tgcJgj13FItftGrF77i7zMYyf-Rxxxxx"
}
- where
1_XlY8tgcJgj13FItftGrF77i7zMYyf-Rxxxxx
is the file id of the Google Docs file where I want to create a script
- Include your script in your project using projects.updateContent
Sample Request Body:
Note: You can replace the timezone in appsscript.json file
{
"files": [
{
"name": "appsscript",
"type": "JSON",
"source": "{\n \"timeZone\": \"Asia/Manila\",\n \"dependencies\": {\n },\n \"exceptionLogging\": \"STACKDRIVER\",\n \"runtimeVersion\": \"V8\",\n \"oauthScopes\": [\n \"https://www.googleapis.com/auth/documents.currentonly\"\n ]\n}"
},
{
"name": "Code",
"type": "SERVER_JS",
"source": "function onOpen(e) {\n // Add a custom menu to the spreadsheet.\n DocumentApp.getUi() // Or DocumentApp, SlidesApp, or FormApp.\n .createMenu('Custom Menu')\n .addItem('Bold Keywords', 'boldKeywords')\n .addToUi();\n}\n\nfunction boldKeywords() {\n // Words that will be put in bold:\n var keywords = [\"end\", \"proc\", \"fun\"];\n\n var document = DocumentApp.getActiveDocument();\n var body = document.getBody();\n\n var Style = {};\n Style[DocumentApp.Attribute.BOLD] = true;\n\n for (j in keywords) {\n\n var found = body.findText(keywords[j]);\n\n while(found != null) {\n var foundText = found.getElement().asText();\n var start = found.getStartOffset();\n var end = found.getEndOffsetInclusive();\n foundText.setAttributes(start, end, Style)\n found = body.findText(keywords[j], found);\n }\n }\n\n}",
"functionSet": {
"values": [
{
"name": "onOpen"
},
{
"name": "boldKeywords"
}
]
}
}
]
}
Output:
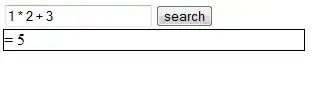
- A custom menu was added in the Google Doc file
If you open the script editor:
Code.gs
function onOpen(e) {
// Add a custom menu to the spreadsheet.
DocumentApp.getUi() // Or DocumentApp, SlidesApp, or FormApp.
.createMenu('Custom Menu')
.addItem('Bold Keywords', 'boldKeywords')
.addToUi();
}
function boldKeywords() {
// Words that will be put in bold:
var keywords = ["end", "proc", "fun"];
var document = DocumentApp.getActiveDocument();
var body = document.getBody();
var Style = {};
Style[DocumentApp.Attribute.BOLD] = true;
for (j in keywords) {
var found = body.findText(keywords[j]);
while(found != null) {
var foundText = found.getElement().asText();
var start = found.getStartOffset();
var end = found.getEndOffsetInclusive();
foundText.setAttributes(start, end, Style)
found = body.findText(keywords[j], found);
}
}
}
appsscript.json
{
"timeZone": "Asia/Manila",
"dependencies": {
},
"exceptionLogging": "STACKDRIVER",
"runtimeVersion": "V8",
"oauthScopes": [
"https://www.googleapis.com/auth/documents.currentonly"
]
}