get_rect()
returns a pygame.Rect
object. A pygame.Rect
stores a position and a size. It is always axis aligned and cannot represent a rotated rectangle.
Use pygame.math.Vector2.rotate()
to compute the corner points of the rotated rectangle. orig_image
is the image before it is roatated:
rect = orig_image.get_rect(center = (self.x, self.y))
pivot = pygame_math.Vector2(self.x, self.y)
p0 = (pygame.math.Vector2(rect.topleft) - pivot).rotate(-angle) + pivot
p1 = (pygame.math.Vector2(rect.topright) - pivot).rotate(-angle) + pivot
p2 = (pygame.math.Vector2(rect.bottomright) - pivot).rotate(-angle) + pivot
p3 = (pygame.math.Vector2(rect.bottomleft) - pivot).rotate(-angle) + pivot
Use pygame.draw.lines()
to draw the rotated rectangle:
pygame.draw.lines(screen, (255, 255, 0), True, [p0, p1, p2, p3], 3)
See also How do I rotate an image around its center using PyGame? and How can you rotate an image around an off center pivot in PyGame.
Minimal example:
repl.it/@Rabbid76/PyGame-RotatedRectangle
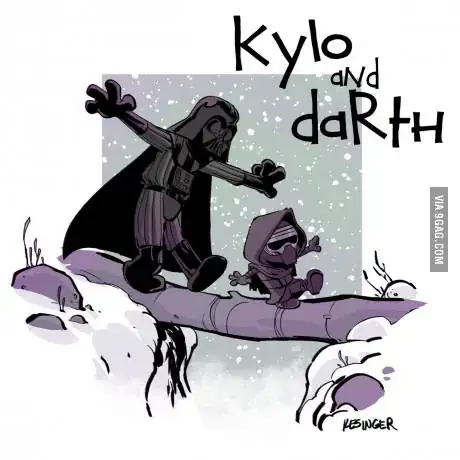
import pygame
pygame.init()
window = pygame.display.set_mode((400, 400))
font = pygame.font.SysFont(None, 50)
clock = pygame.time.Clock()
orig_image = font.render("rotated rectangle", True, (255, 0, 0))
angle = 30
rotated_image = pygame.transform.rotate(orig_image, angle)
def draw_rect_angle(surf, rect, pivot, angle):
pts = [rect.topleft, rect.topright, rect.bottomright, rect.bottomleft]
pts = [(pygame.math.Vector2(p) - pivot).rotate(-angle) + pivot for p in pts]
pygame.draw.lines(surf, (255, 255, 0), True, pts, 3)
run = True
while run:
clock.tick(60)
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
window.fill(0)
window_center = window.get_rect().center
window.blit(rotated_image, rotated_image.get_rect(center = window_center))
rect = orig_image.get_rect(center = window_center)
draw_rect_angle(window, rect, window_center, angle)
pygame.display.flip()
pygame.quit()
exit()