I post my answer originally on Github: https://github.com/matthewwithanm/django-imagekit/issues/444
But, I want to share it right here. I think it can help you and someone else.
So, answering your question: yes, it is possible to preview your image before saving it using the Django admin app.
Here are the steps:
- Define a custom field in the model that has the image that you want to preview (models.py):
from django.contrib.admin.decorators import display
from django.db.models import Model
from django.db.models.fields.files import ImageField
from django.template.loader import get_template
class MyModel(Model):
my_image_field = ImageField()
# This is our new field. It renders a preview of the image before and post save.
@display(description='Preview')
def my_image_thumbnail(self):
return get_template('my_image_thumbnail_template.html').render({
'field_name': 'my_image_field',
'src': self.my_image_field.url if self.my_image_field else None,
})
- Make this new field available in the admin config of the app (admin.py):
from django.contrib import admin
from .models import MyModel
admin.site.register(MyModel, fields=('my_image_thumbnail', 'image'), readonly_fields=('my_image_thumbnail',))
- Define a html template for that new field (my_image_thumbnail_template.html):
<!-- This template is reusable, so you don't need to create one for each field that you want a thumbnail -->
<img
<!--
If there's no image, for example, when we are adding a new instance,
we can fill the empty image preview with a placeholder image.
If want to get this placeholder image URL from your static files,
must load their URL in the `MyModel.my_image_thumbnail` method.
Here is a thread that shows how to do it: https://stackoverflow.com/questions/11721818
-->
src="{% if src %}{{ src }}{% else %}http://atrilco.com/wp-content/uploads/2017/11/ef3-placeholder-image.jpg{% endif %}"
id="image-thumbnail-{{ field_name }}"
>
<script>
// Vainilla javascript (Babel defaults, not ie 11, not ie_mob 11)
// This function adds an event listener over the input image field (`my_field_image`).
// If the user edits that field, the preview image will be updated.
function changeThumbnail() {
const fieldName = "{{ field_name }}";
document
.getElementById(`id_${fieldName}`)
.addEventListener("change", function (e) {
const reader = new FileReader();
reader.onload = function (e) {
document.getElementById(`image-thumbnail-${fieldName}`).src = e.target.result;
};
reader.readAsDataURL(this.files[0]);
});
}
// This function executes a callback when the document is ready.
// https://stackoverflow.com/questions/9899372
//
// Is this required?
// No, but, in most cases, the image preview is rendered above the input field,
// as we do in the `admin.py` module.
// In that case, need to wait for the document to be ready before adding the
// event listener defined in `changeThumbnail`.
function docReady(fn) {
if (document.readyState === "complete" || document.readyState === "interactive") {
setTimeout(fn, 1);
} else {
document.addEventListener("DOMContentLoaded", fn);
}
}
docReady(changeThumbnail);
</script>
Result
New model instance without image
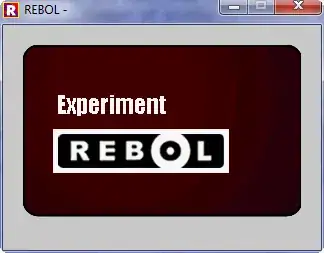
New model instance with image
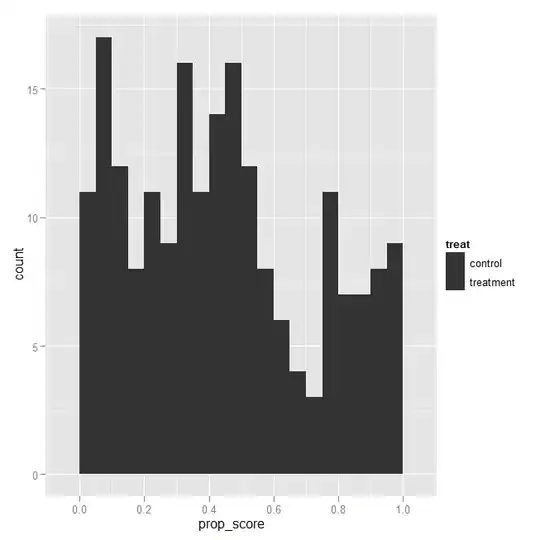
For the curious: I use django-jazzmin (custom Django admin theme)