I am trying to loop through a list containing numbers only. For each loop, I convert the column from char to numeric, and then I attempt to plot it. A basic example of my code is:
library(ggtree)
library(treeio)
library(tidyverse)
library(ggnewscale)
library(ggtreeExtra)
library(argparse)
library(RColorBrewer)
library(rlist)
library(stringr)
tree <- read.tree("/...") #PLEASE REPLACE THIS WITH THE LOCATION TO 'tree_newick.nwk'
tipcategories = read.csv("....", # PLEASE REPLACE THIS WITH THE LOCATION TO 'plot.tsv'
sep = " ",
header = TRUE,
stringsAsFactors = FALSE)
dd = as.data.frame(tipcategories)
p <- ggtree(tree) + ylim(-1, NA) + theme_tree2()
p <- p %<+% dd + geom_tiplab(size=1)
n <- 60
qual_col_pals = brewer.pal.info[brewer.pal.info$category == 'qual',]
col_vector = unlist(mapply(brewer.pal, qual_col_pals$maxcolors,
rownames(qual_col_pals)))
columns = c("Column1", "Column2")
for (col in columns) {
p <- p + new_scale_fill()
dd[[col]] <- as.numeric(as.character(dd[[col]]))
p <- p + geom_fruit(geom=geom_tile, mapping=aes(fill=dd[[col]]), width=2, offset=0.05) +
scale_fill_continuous(name=col, low='blue', high='red')
}
p <- p + theme(legend.text = element_text(size = 5), legend.key.size = unit(0.3, 'cm'))
ggsave("....") # PLEASE REPLACE THIS WITH WHERE YOU WANT TO SAVE IT
The tree data is (please put in file and replace filename with dots in read tree):
(((((((A:4,B:4):6,C:5):8,D:6):3,E:21):10,((F:4,G:12):14,H:8):13):13,((I:5,J:2):30,(K:11,L:11):2):17):4,M:56);
The metadata file (please put in file and replace filename with dots in read.csv):
Accession1 Column1 Column2
A 10 130
B 20 120
C 30 110
D 40 100
E 50 90
F 60 80
G 70 70
H 80 60
I 90 50
J 100 40
K 110 30
L 120 20
M 130 10
The above works fine for just one columns, however, when trying to plot 2 columns, the second column always overwrites the first column, and the first column ends up looking exactly the same as the second column. The below image shows the result of running the program normally.
The first column (column1) is actually supposed to look like this:
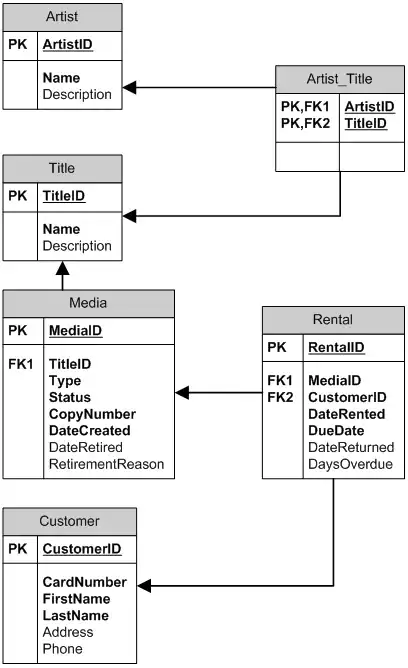
Could anyone provide help as to how to fix this?