- I have 4 red markers inside an image, I'm detecting their contours and getting a rectangular coordinates
[x0,y0,w,h]
.
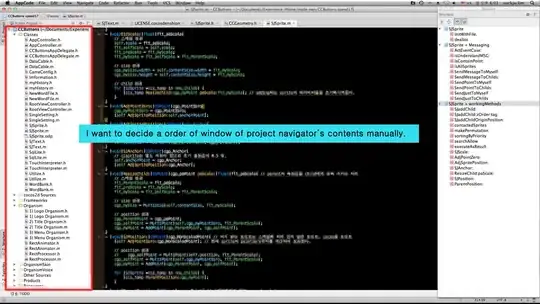

- my goal is to identify the corners in this sequence:
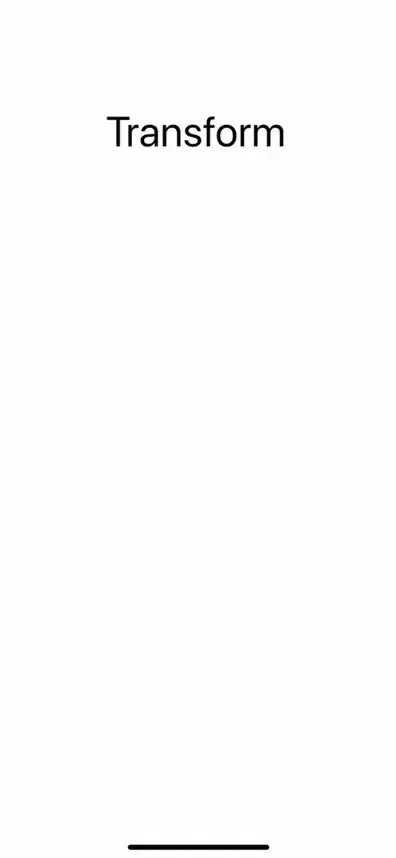
- my problem is when I apply this cool answer I face a problem sometimes because of the sorting according to
Y
values is not always correct as you can see here:
import numpy as np
import cv2
boundRectsList = [(282, 236, 33, 44), (432, 235, 35, 46), (432, 68, 37, 44), (282, 68, 34, 46)]
# Sort the list based on ascending y values:
boundRectsSorted = sorted(boundRectsList, key=lambda x: x[1])
maskCopy = 255*np.ones((512,768,3), dtype=np.uint8)
# Rectangle dictionary:
# Each entry is an index of the currentRect list
# 0 - X, 1 - Y, 2 - Width, 3 - Height
# Additionally: -1 is 0 (no dimension):
pointsDictionary = {0: (2, 3),
1: (-1, 3),
2: (2, -1),
3: (-1, -1)}
# Store center rectangle coordinates here:
centerRectangle = [None]*4
# Process the sorted rects:
rectCounter = 0
for i in range(len(boundRectsSorted)):
# Get sorted rect:
currentRect = boundRectsSorted[i]
# Get the bounding rect's data:
rectX = currentRect[0]
rectY = currentRect[1]
rectWidth = currentRect[2]
rectHeight = currentRect[3]
# Draw sorted rect:
cv2.rectangle(maskCopy, (int(rectX), int(rectY)), (int(rectX + rectWidth),
int(rectY + rectHeight)), (0, 255, 0), 5)
# Get the inner points:
currentInnerPoint = pointsDictionary[i]
borderPoint = [None]*2
# Check coordinates:
for p in range(2):
# Check for '0' index:
idx = currentInnerPoint[p]
if idx == -1:
borderPoint[p] = 0
else:
borderPoint[p] = currentRect[idx]
# Draw the border points:
color = (0, 0, 255)
thickness = 2
centerX = rectX + borderPoint[0]
centerY = rectY + borderPoint[1]
radius = 10
cv2.circle(maskCopy, (centerX, centerY), radius, color, thickness)
# Mark the circle
org = (centerX - 5, centerY + 5)
font = cv2.FONT_HERSHEY_SIMPLEX
cv2.putText(maskCopy, str(rectCounter), org, font,
2, (0, 0, 0), 2, cv2.LINE_8)
# Store the coordinates into list
if rectCounter == 0:
centerRectangle[0] = centerX
centerRectangle[1] = centerY
else:
if rectCounter == 1:
centerRectangle[2] = centerX - centerRectangle[0]
else:
if rectCounter == 2:
centerRectangle[3] = centerY - centerRectangle[1]
# Increase rectCounter:
rectCounter += 1
cv2.namedWindow("Sorted Rects", cv2.WINDOW_NORMAL)
cv2.imshow("Sorted Rects", maskCopy)
cv2.waitKey(0)
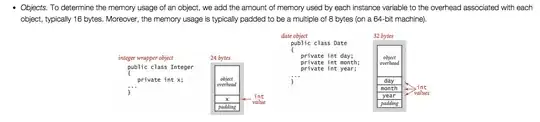
Is there a proper way to get the upper sequence of the internal corners? thanks in advance.