By making a static property:
public static Double TimeLeft { get; set; }
This is if you want to Publicliy accessable from your entire context, if you want it private, just change public
to private
.
Just a side note, the built in Timer
doesn't support polling for the remaining time until the next elapse. Either you decrease TimeLeft
in each Elapse
-event on the 1sec timer or you can have a look at this.
Edit
Here is one way to do it with one timer, first I declare two properties and one constant field that I use, don't bother that they are static, it's just easier to run it as a console application this way.
public static Timer SystemTimer { get; set; }
public static double Elapsed { get; set; }
private const double CycleInterval = 1000;
Then in my Main
-method I have the following to initiate my Timer
SystemTimer = new Timer();
SystemTimer.Interval = CycleInterval;
SystemTimer.Enabled = true;
SystemTimer.Elapsed += Cycle;
SystemTimer.Start();
Having this, the Cycle
-event handler can look like this:
static void Cycle(object sender, ElapsedEventArgs e)
{
Elapsed += CycleInterval;
if ((Elapsed%5000) == 0.0)
{
Console.WriteLine("5 sec elapsed!");
// Do stuff each 5 sec
}
if ((Elapsed % 10000) == 0.0)
{
Console.WriteLine("10 sec elapsed!");
// Do stuff each 10 sec
}
Console.WriteLine("Elapsed: {0}", Elapsed);
}
You could also have Elapsed
being a TimeSpan
, but you can refactor this as you like.
Here's my complete source code that I used:
using System;
using System.IO;
using System.Timers;
namespace ConsoleApplication5
{
class Program
{
public static Timer SystemTimer { get; set; }
public static double Elapsed { get; set; }
private const double CycleInterval = 1000;
static void Main(string[] args)
{
SystemTimer = new Timer();
SystemTimer.Interval = CycleInterval;
SystemTimer.Enabled = true;
SystemTimer.Elapsed += Cycle;
SystemTimer.Start();
while (true) ;
}
static void Cycle(object sender, ElapsedEventArgs e)
{
Elapsed += CycleInterval;
if ((Elapsed%5000) == 0.0)
{
Console.WriteLine("5 sec elapsed!");
// Do stuff each 5 sec
}
if ((Elapsed % 10000) == 0.0)
{
Console.WriteLine("10 sec elapsed!");
// Do stuff each 10 sec
}
Console.WriteLine("Elapsed: {0}", Elapsed);
}
}
}
And this is what it looks like when I run it:
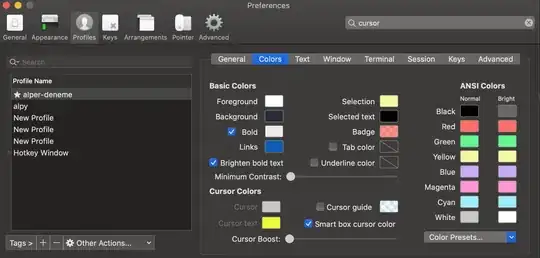