I managed to get the whole page, without iterations, keys or page downs or the lambda function using code from here and only changing the screen size dimensions.
Trouble is, for your webpage at least, the width has to be re-adjusted (expanded) to take the picture of the entire header, and even then it doesn't take the full width and ends up looking stitched together.
The header width is problem in simple selenium-only code.
(on my system):
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_argument('--headless')
chrome_options.add_argument("--window-size=3200x20800") # ANYTHING MORE THAN 3200 width my pycharm cant cope (Rendering error)
driver = webdriver.Chrome(options=chrome_options, executable_path=r"C:\Users\User\Documents\chromedriver_win32\chromedriver.exe") # webdriver.Chrome(options=options)
outFileName = (r'D:\08102020 Random Work\NewFolder4PythonOut')
driver.maximize_window()
URL = "http://stevens.ekkel.ai"
driver.get(URL) #time.sleep(0.5)
#driver.maximize_window()
driver.get_screenshot_as_file(outFileName+"/"+"capture4.png")
Also, That --window-size=3200x20800")
size I the most I could make it on my machine (limited ram space left) before Pycharm wouldn't run or inform me in error message of rendering problems. But even if I could run entire page maximum, the screen shot would look like this , so I recommend you use @Libin Thomas pytest code.
My chrome (deliberately downgraded to chrome 89 as webdriver for chrome 90 doesn't exist yet or I could find), also in a very highly markedup authoritative SO thread (here, here or elsewhere - I've read so much but haven't found a simple generic solution for us) and I [mistake it? or take it? that], as one of the highly marked answers in SO says, Chrome Webdriver doesn't have a "take whole webpage screenshot" function yet, as you can see in the picture, but FireFox does (which I don't want to use, cant and have deleted. RAM)
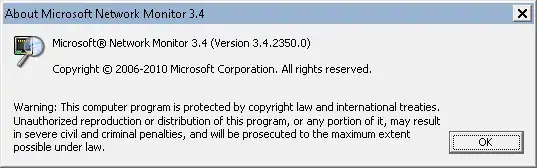
EDIT 18:20 pm
After doing all that , and a bit more, it dawned on me that I was looking at it the wrong way. ZOOM is what I needed.
I did manage to get a screen shot of the whole thing, clearly, in one go, using driver.execute_script("document.body.style.zoom='10%'")
after driver opens the page.
(its helped me understand why even your first attempt, and my successful page down scrolling one, our headers appeared stretched and where narrower than the rest of the body).
The true header is huge compared to the rest, but atleast the screen shot is real and not stretched, or only the middle section of it, Its the whole thing with the real as-is header.
(although probably not what you wanted).
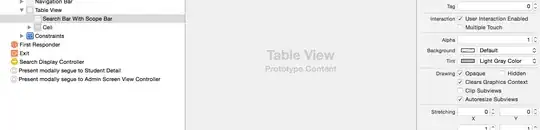
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
chrome_options = Options() #
chrome_options.add_argument('--headless')
chrome_options.headless = True
#https://stackoverflow.com/questions/56201707/how-to-take-screenshot-of-youtube-page-without-opening-the-browser-in-python-or
#chrome_options.add_argument("--window-size=4000x5800")
driver = webdriver.Chrome(options=chrome_options, executable_path=r"C:\Users\User\Documents\chromedriver_win32\chromedriver.exe") # webdriver.Chrome(options=options)
#driver.maximize_window()
outFileName = (r'D:\08102020 Random Work\NewFolder4PythonOut')
URL = "http://stevens.ekkel.ai"
driver.get(URL) #time.sleep(0.5)
#driver.maximize_window()
driver.execute_script("document.body.style.zoom='10%'")
driver.get_screenshot_as_file(outFileName+"/"+"capture5.png")
I hope I've helped in some small way, even if not the way you maybe wanted.