document
is the actual object that is created & HTMLDocument
is used to construct it. You can check it by typing document.constructor
in the console, which will show HTMLDocuement
in the console. JavaScript uses prototypal inheritance & according to this post, internal __proto__
property is used to navigate the prototype inheritance chain.
HTMLDcoument itself is constructed using Function constructor
as any other object in JavaScript. That is why, the following statement evaluates to true
.
HTMLDocument.constructor == Function // true
But HTMLDocument
is intended to use as a extension for Document
interface. So that, any object
of type HTMLDocument
must have access to all the properties of Document
interface as well. In order to maintain that, __proto__
of HTMLDocuemnt
is internally set to the Document
object. That's why HTMLDocument.__proto__
doesn't refer to Function.prototype
.
HTMLDocument.__proto__ == Document // true.
HTMLDocument.__proto__ == Function // false
The code snippet above means, HTMLDocument
object will have access to all the properties from Document
object via its prototype chain directly, not the Function
object. And you must already know, that is for obvious reasons.
That left us with,
HTMLDocument.prototype == document.__proto__ // true
Above statement means, document
object will have access to its parent HTMLDocument's
prototype object, as all instance objects do.
Here is the total inheritance chain for your convenience:
document (actual object)--> HTMLDocument-->Document-->Node-->EventTarget-->Function
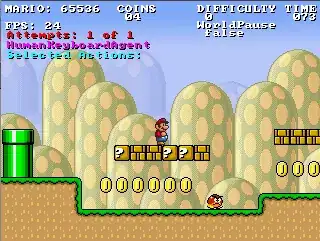