The matrix that is passed as a Hypergraph
constructor should have elements of type Union{Nothing, T}
where T
is some numeric type (e.g. Matrix{Union{Float64,Nothing}}
rather than just be a Matrix{Float64}
.
In SimpleHypegraphs.jl, we use nothing
(rather than 0
) to represent that a vertex does not belong to a hyperedge since in many hypergraph algorithms/applications it is possible for a vertex to belong to a hyper-edge with a zero weight.
Hence you could read your file with the following code (for reproducibility I put the file content into a text variable):
using DataFrames, CSV, SimpleHypergraphs
txt = """0.0, 7.0, 0.0, 0.0
1.3, 2.8, 4.5, 0.0
0.0, 1.3, 3.1, 4.2
1.2, 0.0, 5.6, 5.0"""
df = CSV.read(IOBuffer(txt), DataFrame;header=0)
mat = Matrix{Union{Float64,Nothing}}(df)
This matrix can now easily be used as a Hypergraph
constructor.
julia> h1 = Hypergraph(mat)
4×4 Hypergraph{Float64, Nothing, Nothing, Dict{Int64, Float64}}:
0.0 7.0 0.0 0.0
1.3 2.8 4.5 0.0
0.0 1.3 3.1 4.2
1.2 0.0 5.6 5.0
However, this might be not exactly what you need because zeros (0.0
) represent a situation where vertices belong to hyperedges with zero weights:
draw(h1, HyperNetX)

Hence, you might actually want to convert zeros to nothing
s to represent situation where the vertices do not belong to hyperedges:
h2 = Hypergraph(replace(mat, 0 => nothing))
Now you get what you wanted:
draw(h2, HyperNetX)
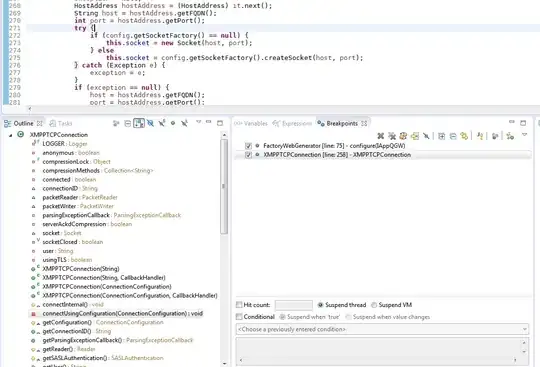