Per the plotly docs on multiple axis graphs:
Note: At this time, Plotly Express does not support multiple Y axes on a single figure. To make such a figure, use the make_subplots()
function in conjunction with graph objects as documented below.
Here is a solution that uses the more fully-featured graph_objects
:
import pandas as pd
import plotly.graph_objects as go
from plotly.subplots import make_subplots
data = [['A',100,880],['B ',50,450],['C',25,1200]]
df = pd.DataFrame(data,columns=['Letter','Column1','Column2'])
fig = make_subplots(specs=[[{"secondary_y": True}]])
fig.add_trace(
go.Scatter(x=df['Letter'], y=df['Column1'], name="Column1", mode="lines"),
secondary_y=True
)
fig.add_trace(
go.Bar(x=df['Letter'], y=df['Column2'], name="Letter"),
secondary_y=False
)
fig.update_xaxes(title_text="Letter")
# Set y-axes titles
fig.update_yaxes(title_text="Column2", secondary_y=False)
fig.update_yaxes(title_text="Column1", secondary_y=True)
fig.show()
which gives
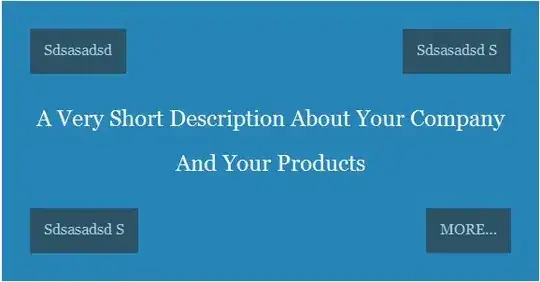
As shown on the docs page linked above, there are multiple ways to achieve this which give you quite a bit of flexibility.
Though not officially supported, if you feel like you really need express
you could try the answer offered on this SO post.