Unfortunely this is not really a case that ActiveRecord assocations really can handle that well. Assocations just link a single foreign key on one model to a primary key on another model and you can't just chain them together.
If you wanted to join both you would need the following JOIN clause:
JOINS matches ON matches.mentor_id = entities.id
OR matches.mentee_id = entities.id
You just can't get that as assocations are used in so many ways and you can't just tinker with the join conditons. To create a single assocation that contains both categories you need a join table:
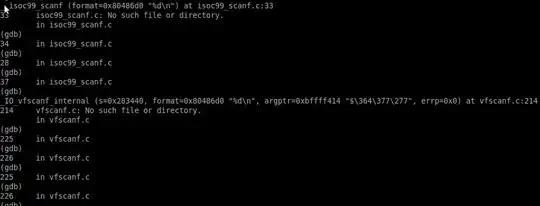
class Entity < ApplicationRecord
# Easy as pie
has_many :matchings
has_many :matches, through: :matchings
# this is where it gets crazy
has_many :matchings_as_mentor,
class_name: 'Matching',
->{ where(matchings: { role: :mentor }) }
has_many :matches_as_mentor,
class_name: 'Match',
through: :matchings_as_mentor
has_many :matchings_as_mentee,
class_name: 'Matching',
->{ where(matchings: { role: :mentee }) }
has_many :matches_as_mentee,
class_name: 'Match',
through: :matchings_as_mentor
end
class Matching < ApplicationRecord
enum role: [:mentor, :mentee]
belongs_to :entity
belongs_to :match
validates_uniqueness_of :entity_id, scope: :match_id
validates_uniqueness_of :match_id, scope: :role
end
class Match < ApplicationRecord
# Easy as pie
has_many :matchings
has_many :entities, through: :matching
# this is where it gets crazy
has_one :mentor_matching,
class_name: 'Matching',
->{ where(matchings: { role: :mentee }) }
has_one :mentor, through: :mentor_matching, source: :entity
has_one :mentee_matching,
class_name: 'Matching',
->{ where(matchings: { role: :mentor }) }
has_one :mentee, through: :mentor_matching, source: :entity
end
And presto you can reap the rewards of having a homogenous association:
entities = Entity.includes(:matches)
entities.each do |e|
puts e.matches.order(:name).inspect
end
It is a lot more complex though and you need validations and constraints to ensure that a match can only have one mentor and mentee. its up to you to evaluate if its worth it.
The alternative is doing somehing like:
Match.where(mentor_id: entity.id)
.or(Match.where(mentee_id: entity.id))
Which cannot does not allow eager loading so it would cause a N+1 query issue if you are displaying a list of entites and their matches.