The code for best fit line of a scatter plot has already been answered here.
And for the other subplots, you can just change the index from ax[0, 0]
to other index like ax[0, 1]
. Because you are creating a 2x2 subplots, the indices are ax[0,0]
, ax[0,1]
, ax[1,0]
, and ax[1,1]
.
So implementing for multiple subplots, would be:
import numpy as np
import matplotlib.pyplot as plt
x = np.random.normal(0, 1, 15)
y1 = np.random.normal(0, 1, 15)
y2 = np.random.normal(0, 1, 15)
y3 = np.random.normal(0, 1, 15)
y4 = np.random.normal(0, 1, 15)
fig, ax = plt.subplots(2,2, figsize=(20,18))
ax[0,0].scatter(x, y1, color='tab:blue')
ax[0,0].plot(np.unique(x), np.poly1d(np.polyfit(x, y1, 1))(np.unique(x)))
ax[0,0].set_xlabel('Title One', fontsize=15)
ax[0,0].set_ylabel('Title Two', fontsize=15)
ax[0,1].scatter(x, y2, color='tab:blue')
ax[0,1].plot(np.unique(x), np.poly1d(np.polyfit(x, y2, 1))(np.unique(x)))
ax[0,1].set_xlabel('Title One', fontsize=15)
ax[0,1].set_ylabel('Title Two', fontsize=15)
ax[1,0].scatter(x, y3, color='tab:blue')
ax[1,0].plot(np.unique(x), np.poly1d(np.polyfit(x, y3, 1))(np.unique(x)))
ax[1,0].set_xlabel('Title One', fontsize=15)
ax[1,0].set_ylabel('Title Two', fontsize=15)
ax[1,1].scatter(x, y4, color='tab:blue')
ax[1,1].plot(np.unique(x), np.poly1d(np.polyfit(x, y4, 1))(np.unique(x)))
ax[1,1].set_xlabel('Title One', fontsize=15)
ax[1,1].set_ylabel('Title Two', fontsize=15)
plt.show()
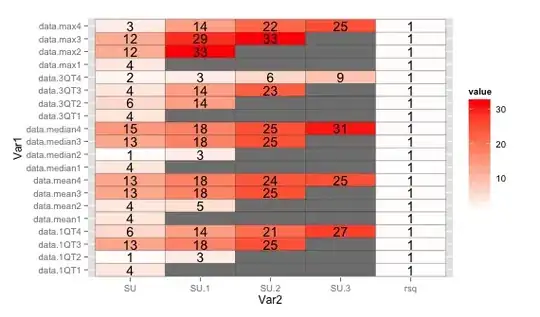