Here is an idea to create a plot that looks a bit similar. Starting from 300 random centers, a kde kernel is calculated with a very small bandwidth. The gaussian_kde
function places gaussian bell shapes over each of the random centers and sums them.
The following code is a bit slow, because the surface is calculated and plotted over 200x200 positions. For scaling down the z-axis, the code from this post is borrowed.
import matplotlib.pyplot as plt
from scipy.stats import gaussian_kde
import numpy as np
x = np.linspace(0, 251, 200)
y = np.linspace(0, 12, 200)
centers_x = np.random.uniform(x[0], x[-1], 300)
centers_y = np.random.uniform(y[0], y[-1], 300)
centers = np.vstack([centers_x, centers_y])
kernel = gaussian_kde(centers, bw_method=0.09)
X, Y = np.meshgrid(x, y)
positions = np.vstack([X.ravel(), Y.ravel()])
Z = np.reshape(kernel(positions).T, X.shape)
fig = plt.figure(figsize=(10, 6))
ax = plt.axes(projection='3d')
surf = ax.plot_surface(X, Y, Z, cmap="hot", linewidth=0, rcount=200, ccount=200)
ax.get_proj = lambda: np.dot(Axes3D.get_proj(ax), np.diag([1, 1, 0.1, 1]))
ax.set_zticks([])
plt.show()
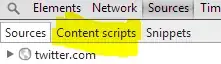