You are incorrectly comparing String
s, see How do I compare Strings in Java?. Furthermore, the logic you currently applied for the counting of the rows is faulty.
Currently you are not narrowing down the rows with only "Softbound". You would still be displaying the complete row count if "Softbound" was matched with a retrieved value.
To fix your logic, you need to create some sort of counter, loop through the rows as you do, correctly compare the values (with equals or equalsIgnoreCase), increment the counter on each found row, and then after you looped through all rows, display the found amount.
Working example that fixes your used approach:
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> buildGui());
}
private static void buildGui() {
JFrame frame = new JFrame();
JPanel panel = new JPanel();
String[] header = { "ISBN", "Title", "Type" };
Object[][] data = { { 1111, "Title1", "Softbound" }, { 2222, "Title2", "Softbound" }, { 3333, "Title3", "Hardbound" } };
JTable table = new JTable(data, header);
JLabel label = new JLabel("Found result:");
JLabel labelAmount = new JLabel();
JComboBox<String> comboBox = new JComboBox<>(new String[] { "Hardbound", "Softbound" });
JScrollPane scrollPane = new JScrollPane(table);
JButton btn = new JButton("Get amount");
btn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int amount = 0;
for (int i = 0; i < table.getRowCount(); i++) {
if (table.getValueAt(i, 2).equals(comboBox.getSelectedItem())) {
amount++;
}
}
labelAmount.setText("" + amount);
}
});
frame.add(scrollPane, BorderLayout.CENTER);
panel.add(comboBox);
panel.add(btn);
panel.add(label);
panel.add(labelAmount);
frame.add(panel, BorderLayout.SOUTH);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
Result:
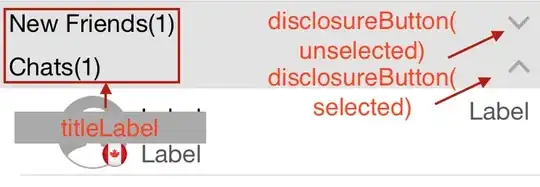
Sidenote:
If you want to modify the displayed values shown in your JTable
, especially by sorting and filtering, this can be done with some useful tools as shown in the tutorial How to use Tables - Sorting and Filtering. Have a closer look at the TableFilterDemo
and the RowFilter
.