Since you did not provide a excel-file, i cooked up a file based on your images provided..
It looks like this

library(tidyverse)
library(tidyxl)
library(readxl)
library(data.table)
library(unpivotr)
file_to_read <- "./testdata.xlsx"
# Get all names of sheets in the file
sheet_names <- readxl::excel_sheets(file_to_read)
# Loop through sheets
L <- lapply(sheet_names, function(x) {
all_cells <-
tidyxl::xlsx_cells(file_to_read, sheets = x) %>%
dplyr::select(sheet, row, col, data_type, character, numeric)
# Cells with the actual data
cells_data <-
dplyr::filter(all_cells, row >= 3, col >= 3) %>%
dplyr::transmute(row, col, sheet = sheet, value = numeric)
# Select the headers
person.number.up <-
dplyr::filter(all_cells, row == 1) %>%
dplyr::select(row, col, person.number.up = character)
person.type.up <-
dplyr::filter(all_cells, row == 2) %>%
dplyr::select(row, col, person.type.up = character)
person.number.left <-
dplyr::filter(all_cells, col == 1) %>%
dplyr::select(row, col, person.number.left = character)
person.type.left <-
dplyr::filter(all_cells, col == 2) %>%
dplyr::select(row, col, person.type.left = character)
#put together
final.df <- cells_data %>%
unpivotr::enhead(person.number.up, "up-ish") %>%
unpivotr::enhead(person.type.up, "up-ish") %>%
unpivotr::enhead(person.number.left, "left-ish") %>%
unpivotr::enhead(person.type.left, "left-ish") %>%
dplyr::select(-(1:2))
})
# Put together in a data.table
DT <- data.table::rbindlist(L, use.names = TRUE)
# Cast to wide, summing values in the process
ans <- dcast(DT, person.number.left + person.type.left ~ person.number.up + person.type.up,
value.var = "value",
fun.aggregate = sum, na.rm = TRUE)
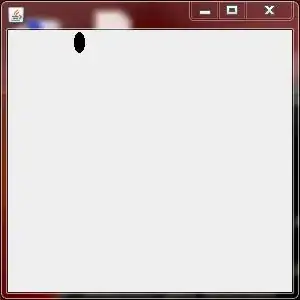