Ok, based on the comments from @Alex & @James_D, now it is quite clear that you cannot control radius for a background-image. But if you are very keen to get the desired behavior(get curved edges to background image) you can set shape to the node to mimic like a background-radius is applied on the background image.
You can build the dynamic shape path based on your required radius and apply the style on the node.
Please check the below demo code. If you have fixed size node, you can directly call updateShape
method, if the node size changes, you can call this on width/height listener. Also check the image for a quick preview of how the shape path is built.
[Update :] As per the comments from @Slaw, included a much simpler alternate approach of setting Rectangle as shape and updating its dimensions.
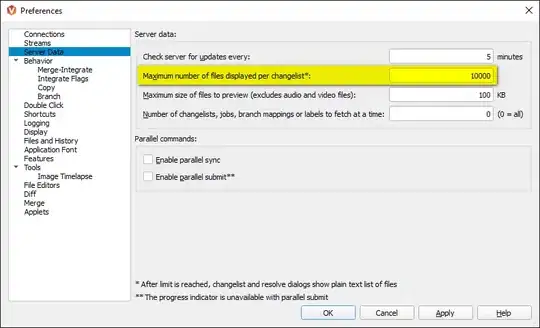
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
public class BackgroundImageRadiusDemo extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
double radius = 20;
StackPane signUp = new StackPane();
signUp.getStyleClass().add("signUp"); // In the .css file it sets -fx-background-
// APPROACH #1 : Using Rectangle as shape
Rectangle r = new Rectangle(30, 30);
r.setArcHeight(radius);
r.setArcWidth(radius);
signUp.setShape(r);
signUp.widthProperty().addListener(p -> r.setWidth(signUp.getWidth()));
signUp.heightProperty().addListener(p -> r.setHeight(signUp.getHeight()));
// APPROACH #2 : Using SVG path as shape
// signUp.widthProperty().addListener(p -> updateShape(signUp));
// signUp.heightProperty().addListener(p -> updateShape(signUp));
StackPane root = new StackPane(signUp);
root.setPadding(new Insets(10));
Scene scene = new Scene(root, 300, 250);
scene.getStylesheets().add(this.getClass().getResource("cssFile.css").toExternalForm());
primaryStage.setTitle("BackgroundImage Radius");
primaryStage.setScene(scene);
primaryStage.show();
}
private void updateShape(StackPane signUp) {
double r = 20; // BACKGROUND IMAGE RADIUS
double w = signUp.getWidth();
double h = signUp.getHeight();
String s = "-fx-shape:\"M " + r + " 0 " + //p1
" L " + (w - r) + " " + "0" + //p2
" Q " + w + " 0 " + w + " " + r + // p3,p4
" L " + w + " " + (h - r) + //p5
" Q " + w + " " + h + " " + (w - r) + " " + h + //p6,p7
" L " + r + " " + h + //p8
" Q 0 " + h + " 0 " + (h - r) + //p9,p10
" L 0 " + r + //p11
" Q 0 0 " + r + " 0 Z\";"; //p0,p1
System.out.println(s);
signUp.setStyle(s);
}
}
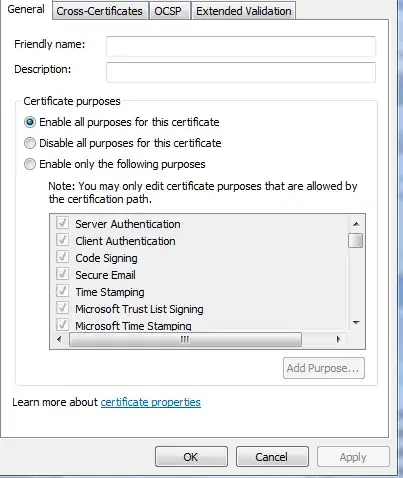