Another way to achieve what you want without looping.
LOGIC:
- Identify the worksheet you are going to work with.
- Remove any autofilter.
- Check if the column
D
has 0
anywhere.
- Find last row in column
D
. Better than hardcoding D4005
- Construct your range.
- Filter column
D
based on 0
.
- Identify the filtered range.
- Clear the cells in 2 columns without looping.
CODE:
Is this what you are trying? I have commented the code so you should not have a problem understanding it but if you do them simply ask :)
Option Explicit
Sub Sample()
Dim ws As Worksheet
Dim lRow As Long
Dim FilteredRange As Range
'~~> Set this to the relevant sheet
Set ws = Sheet1
With ws
'~~> Remove any filters
.AutoFilterMode = False
'~~> Find last row
lRow = .Range("D" & .Rows.Count).End(xlUp).Row
'~~> Check if the column D has 0 anywhere
If Application.WorksheetFunction.CountIf(.Range("D1:D" & lRow), 0) Then
'~~> Filter column D on 0
.Range("D1:D" & lRow).AutoFilter Field:=1, Criteria1:=0
'~~> Identify your range
Set FilteredRange = .AutoFilter.Range
'~~> Clear the range in 1 go!
FilteredRange.Offset(1, 0).Resize(FilteredRange.Rows.Count - 1).Clear
FilteredRange.Offset(1, -1).Resize(FilteredRange.Rows.Count - 1).Clear
End If
'~~> Remove any filters
.AutoFilterMode = False
End With
End Sub
BEFORE:
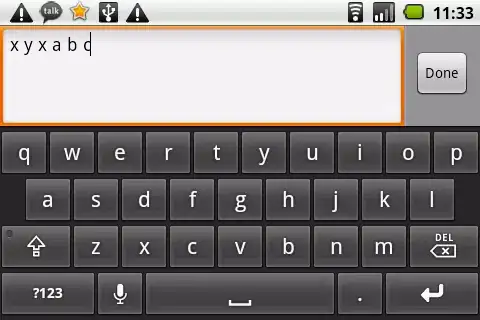
AFTER:
