I think this is what you want:
library(dplyr)
library(ggplot2)
library(tibble)
##----------------------------------------
## Creating a dataset to simulate yours
##----------------------------------------
df <- tibble(
beetle = sample(0:25, 500, replace = TRUE),
loclity_division = sample(c("Municipality", "Village"), 500, replace = T),
)
df$beetle <- ifelse(df$beetle > 1 & df$beetle <= 5, "1-5",
ifelse(df$beetle > 5 & df$beetle <= 10, "6-10",
ifelse(df$beetle > 10 & df$beetle <= 15, "11-15",
ifelse(df$beetle > 15, "Above 15", "0"
))))
##--------------------------------------
## Determine the custom order of levels
##--------------------------------------
df$beetle <- factor(df$beetle, levels = c("0", "1-5", "6-10", "11-15", "Above 15"))
##----------------------
## Plotting by ggplot
##----------------------
df %>%
ggplot(aes(x = beetle, fill = beetle)) +
geom_bar(width = 0.6) +
coord_flip() +
facet_wrap(vars(loclity_division))
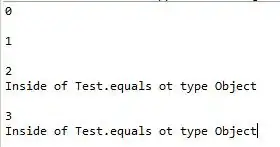