Alright so here is what I came up with:
Create a dummy dataframe (sampled every 5 minutes):
import pandas as pd
import numpy as np
import matplotlib.pylab as plt
import matplotlib.dates as mdates
df = pd.DataFrame(np.random.rand(581,2),index=pd.date_range(start='2020-01-01 00:00:00', end='2020-01-03 00:24:00', freq='5T'))
print(df)
Pandas will automatically perform a tick resolution change assuming regular fequency timeseries data. The x_compact parameter = True will suppress that behavior.
ax = df.plot(x_compat=True)
ax.xaxis.set_major_locator(mdates.HourLocator(interval=4)) #to get a tick every 4 hours
ax.xaxis.set_major_formatter(mdates.DateFormatter('%H:%M')) #format what the labels look like
Edit: I noticed the labels and x ticks were not quite in alignment, this should hopefully fix that:
for label in ax.xaxis.get_ticklabels():
label.set_horizontalalignment('center')
ax.xaxis.set_tick_params(rotation=90) # rotate the x axis labels, if you want
plt.show()
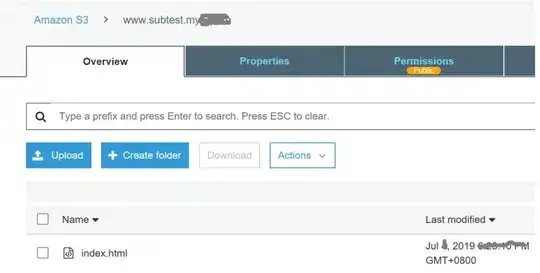
I don't know what exactly your data looks like so hopefully this is enough to go on.
Edit
Alright so after realizing you code uses bar plots I have updated the code. I think you are going to have to use matplotlib in order to plot the bar plots and get the datetime to show up properly and edit the x axis (see this question and this question).
So try to adapt your code to do something similar to as follows:
import random
import pandas as pd
import numpy as np
import matplotlib.pylab as plt
import matplotlib.dates as mdates
random.seed(30)
df = pd.DataFrame({
"Site 1": np.random.rand(577),
"Site 2": np.random.rand(577),})
idx = pd.date_range(start='2020-01-01 00:00:00', end='2020-01-03 00:00:00',freq ='5T')
df = df.set_index(idx)
fig, ax = plt.subplots(figsize=(12,8))
ax.bar(df.index, df['Site 1'], width=0.005)
ax.xaxis.set_major_locator(mdates.HourLocator(interval=4)) #to get a tick every 4 hours
ax.xaxis.set_major_formatter(mdates.DateFormatter('%H:%M'))
ax.tick_params(axis='x', labelrotation = 90)
plt.show()
You will need to set up a subplot here to show both of the plots but this should hopefully get you on the right track. Sorry about the confusion, I am learning these things right there with you!