Adding to the @csalmhof's answer, I think it's to explain here why using boxed
is working.
If you don't use boxed()
method and simply write the following:
Map<Integer, String> map = IntStream.range(0, headerDisplayName.length)
.collect(Collectors.toMap(
Function.identity(),
index -> headerDisplayName[index])
);
Java will have to take index
as of type Object
and there's no implicit conversion that can happen and so you'll get error.
But if you put boxed()
like in the following code, you won't get error:
Map<Integer, String> map = IntStream.range(0, headerDisplayName.length)
.boxed()
.collect(Collectors.toMap(
Function.identity(),
index -> headerDisplayName[index])
);
you're not getting error here because java interprets index
as an Integer
and there's implicit casting happening from Integer
to int
.
A good IDE can help you with this type of explanation. In IntelliJ if you press ctrl
+ space
after keeping your cursor on index
(with Eclipse key-map enabled) you will get the following without boxed()
.
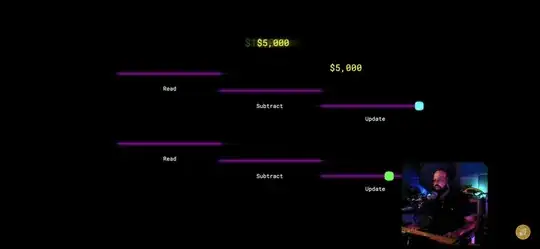
And this is what you get when you've boxed()
placed.
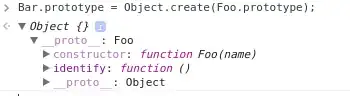
I hope this clarifies why using boxed() is saving you from compilation error. Also, you can use this thing in future to find actual type of parameter in lambda which can be helpful in case cases (one of the case is the one that OP pointed out)