Seaborn's regplot()
can be used to create such a plot. Here is some example code.
from matplotlib import pyplot as plt
import seaborn as sns
import pandas as pd
from io import StringIO
data_str = '''"Car Model" Mileage "Sell Price($)" Age(yrs)
"BMW X5" 69000 18000 6
"BMW X5" 35000 34000 3
"BMW X5" 57000 26100 5
"BMW X5" 22500 40000 2
"BMW X5" 46000 31500 4
"Audi A5" 59000 29400 5
"Audi A5" 52000 32000 5
"Audi A5" 72000 19300 6
"Audi A5" 91000 12000 8
"Mercedez Benz C class" 67000 22000 6
"Mercedez Benz C class" 83000 20000 7
"Mercedez Benz C class" 79000 21000 7
"Mercedez Benz C class" 59000 33000 5'''
df = pd.read_csv(StringIO(data_str), delim_whitespace=True)
fig, axs = plt.subplots(nrows=3, ncols=2, figsize=(12, 10))
sns.regplot(data=df[df["Car Model"] == "BMW X5"], x='Age(yrs)', y='Sell Price($)', ax=axs[0, 0])
sns.regplot(data=df[df["Car Model"] == "BMW X5"], x='Age(yrs)', y='Mileage', ax=axs[0, 1])
axs[0, 0].set_title("BWM X5")
axs[0, 1].set_title("BWM X5")
sns.regplot(data=df[df["Car Model"] == "Audi A5"], x='Age(yrs)', y='Sell Price($)', ax=axs[1, 0])
sns.regplot(data=df[df["Car Model"] == "Audi A5"], x='Age(yrs)', y='Mileage', ax=axs[1, 1])
axs[1, 0].set_title("Audi A5")
axs[1, 1].set_title("Audi A5")
sns.regplot(data=df[df["Car Model"] == "Mercedez Benz C class"], x='Age(yrs)', y='Sell Price($)', ax=axs[2, 0])
sns.regplot(data=df[df["Car Model"] == "Mercedez Benz C class"], x='Age(yrs)', y='Mileage', ax=axs[2, 1])
axs[2, 0].set_title("Mercedez Benz C class")
axs[2, 1].set_title("Mercedez Benz C class")
plt.tight_layout()
plt.show()
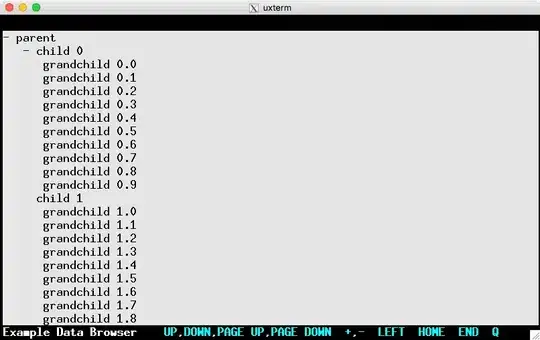
PS: You might want to read about the difference between plt.subplots()
and plt.subplot()
(with and without final s
). For example: What is the difference between drawing plots using plot, axes or figure in matplotlib?.
Via extra variables and for
loops, fewer lines need to repeated. For example, the same code could be written as:
fig, axs = plt.subplots(nrows=3, ncols=2, figsize=(12, 10))
car_models = df["Car Model"].unique()
for ax_row, car_model in zip(axs, car_models):
for ax, y_column in zip(ax_row, ['Sell Price($)', 'Mileage']):
sns.regplot(data=df[df["Car Model"] == car_model], x='Age(yrs)', y=y_column, ax=ax)
ax.set_title(car_model)
plt.tight_layout()
plt.show()
Seaborn also allows to create the full set of subplots in one go, using sns.relplot()
. For this to work, the two y-columns need to be "melted" to create a "long form" dataframe.