This is one potential solution:
library(tidyverse)
dat1 <- tibble(Date = seq.Date(from = as.Date("2015-03-20"),
to = as.Date("2015-03-30"),
length.out = 30),
direction = rep(c("N", "null", "U"), 10),
Detections = sample(x = c(1:5),
size = 30,
replace = TRUE))
dat2 <- dat1 %>%
mutate(Detections = replace(Detections, direction == "null", 0))
ggplot() +
geom_line(data = dat2 %>% filter(direction != "null"),
aes(x = Date, y = Detections,
col = direction), size = 1) +
geom_line(data = dat2 %>% filter(direction == "null"),
aes(x = Date, y = Detections),
show.legend = FALSE, col = "#009E73", size = 1) +
theme_bw(base_size = 16, base_family = "serif") +
theme(panel.grid.major = element_blank(),
panel.grid.minor = element_blank()) +
ggtitle("RM 2015")+
theme(plot.title = element_text(hjust = 0.5)) +
scale_color_manual(name = "Direction",
values = c("#D55E00","#0072B2"),
labels = c("Downstream","Upstream")) +
scale_y_continuous(breaks = seq(0,10,2),
expand = c(0.01,0),
limits = c(0,10))
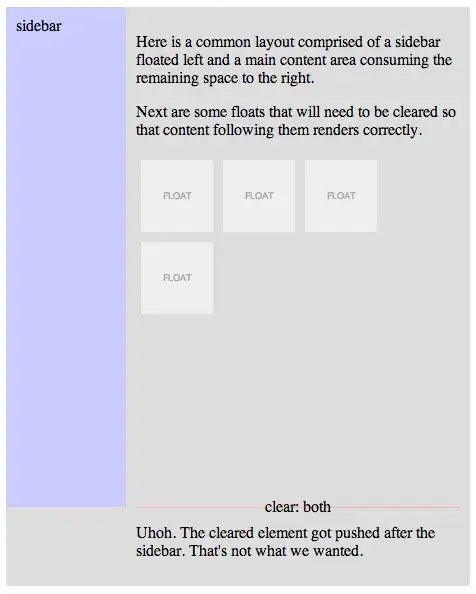
Basically, plot one set of lines (direction == "U" or "N"), then plot the "null" line (direction == "null") but leave it out of the legend. I left out the scale_x_datetime()
in my example, but it shouldn't cause any issues to include it like in your original code.
Edit
I'm afraid I still don't understand what you are trying to achieve. Is this any better?
library(tidyverse)
set.seed(1)
dat1 <- tibble(Date = seq.Date(from = as.Date("2015-03-20"),
to = as.Date("2015-03-31"),
length.out = 30),
direction = rep(c("N", "null", "U"), 10),
Detections = sample(x = c(1:5),
size = 30,
replace = TRUE))
dat2 <- dat1 %>%
mutate(Detections = Detections - 1) %>%
mutate(Detections = replace(Detections, direction == "null", 0))
ggplot() +
geom_line(data = dat2 %>% filter(direction != "null"),
aes(x = Date, y = Detections,
col = direction), size = 1) +
geom_line(data = dat2 %>% filter(direction == "null"),
aes(x = Date, y = Detections),
show.legend = FALSE, col = "#009E73", size = 1) +
theme_bw(base_size = 16, base_family = "serif") +
ggtitle("RM 2015") +
scale_color_manual(name = "Direction",
values = c("#D55E00","#0072B2"),
labels = c("Downstream","Upstream")) +
scale_y_continuous(breaks = seq(0, 10, 2),
expand = c(0.01, 0),
limits = c(0, 10)) +
scale_x_date(date_breaks = "1 day", date_labels = "%d-%m-%Y",
limits = c(as.Date("2015-03-20"), as.Date("2015-03-31"))) +
theme(panel.grid.major = element_blank(),
panel.grid.minor = element_blank(),
plot.title = element_text(hjust = 0.5),
axis.text.x = element_text(angle = 45, hjust = 1, vjust = 0.95),
axis.title.x = element_blank())
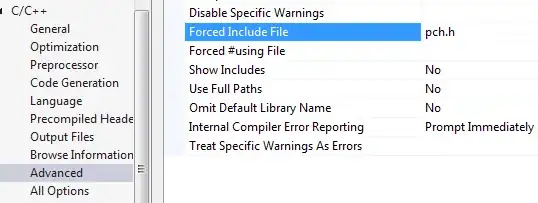