I did a webpage to play with those properties and it's values. It seems the only way to get what is needed in this case, the right green box positioning, is thought empiricism. So this is the code:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<style>
#result { width:2000px; height:1600px; }
#bottomright {
position: fixed;
right: 0px;
bottom: 0px;
width: 64px;
height: 64px;
border: black solid 1px;
}
</style>
<script type="text/javascript">
function ini() {
var Sizes = [
{ method:"window.inner", color:"red", width:window.innerWidth, height:window.innerHeight },
{ method:"document.body.offset", color:"blue", width:document.body.offsetWidth, height:document.body.offsetHeight }
];
var html = "<h2>Methods do get de window size:</h2>";
for(let i=0; i<Sizes.length; i++) {
html += '<p style="color:'+Sizes[i].color+';">'+Sizes[i].method+'</p>';
document.body.appendChild(Box(0, 0, 64, 64, Sizes[i].color));
document.body.appendChild(Box(Sizes[i].width-66, 0, 64, 64, Sizes[i].color));
document.body.appendChild(Box(0, Sizes[i].height-66, 64, 64, Sizes[i].color));
document.body.appendChild(Box(Sizes[i].width-66, Sizes[i].height-66, 64, 64, Sizes[i].color));
}
const size = realSize();
document.body.appendChild(Box(size.width-34, size.height-34, 32, 32, "green"));
html += '<p style="color:green;">Real Size</p>';
document.getElementById("result").innerHTML = html;
}
function Box(x, y, w, h, color) {
const elm = document.createElement("div");
elm.style.border = color+" solid 1px";
elm.style.position = "fixed";
elm.style.left = x.toString()+"px";
elm.style.top = y.toString()+"px";
elm.style.width = w.toString()+"px";
elm.style.height = h.toString()+"px";
return elm;
}
function realSize() {
const elm = document.createElement("div");
elm.style.id = "sizeFinder"; // will look at later
elm.style.border = "0px"; // just in case
elm.style.position = "fixed";
elm.style.right = "0px"; // the real right
elm.style.bottom = "0px"; // the real bottom
elm.style.width = "10px"; // some width, just in case
elm.style.height = "10px"; // some height, just in case
document.body.appendChild(elm);
const box = elm.getBoundingClientRect()
return { width:box.x+10, height:box.y+10 };
}
</script>
<title>Window Size Test</title>
</head>
<body onload="ini();">
<h1>Window Size Test</h1>
<div id="result"></div>
<div id="bottomright"></div>
</body>
</html>
This is the result:
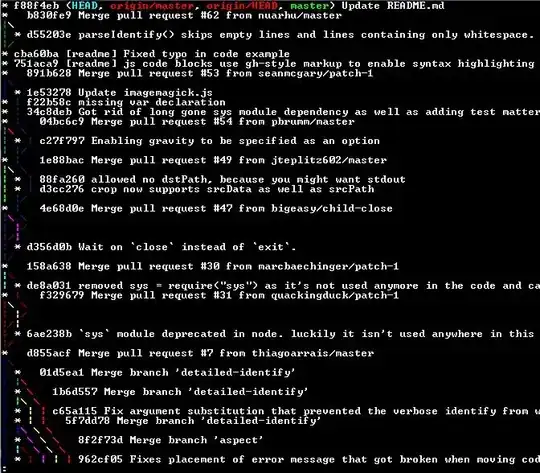
Note that I did the green box with half size to not cover the other boxes. Also, might be a good idea to have an invisible box fixed at the corner to get the actual window size when needed, thought is what I will do.
Please test and share comments, I am still worry if this will work with any browsers and have some retro compatibility.