I assume you simply added small random RGB to your image...
I would leave color as is and try to reduce color intensity by small random amount to darken the image and create a similar form of noise. Just by multiply your RGB color with random number less than one ...
To improve result visual the random value should be with gauss distribution or similar. Here small C++/VCL example:
//$$---- Form CPP ----
//---------------------------------------------------------------------------
#include <vcl.h>
#include <math.h>
#pragma hdrstop
#include "win_main.h"
//---------------------------------------------------------------------------
#pragma package(smart_init)
#pragma resource "*.dfm"
TMain *Main;
Graphics::TBitmap *bmp0,*bmp1;
//---------------------------------------------------------------------------
__fastcall TMain::TMain(TComponent* Owner) : TForm(Owner)
{
// init bmps and load from file
bmp0=new Graphics::TBitmap;
bmp1=new Graphics::TBitmap;
bmp0->LoadFromFile("in.bmp");
bmp0->HandleType=bmDIB;
bmp0->PixelFormat=pf32bit;
bmp1->Assign(bmp0);
ClientWidth=bmp0->Width;
ClientHeight=bmp0->Height;
Randomize();
}
//---------------------------------------------------------------------------
void __fastcall TMain::FormDestroy(TObject *Sender)
{
// free bmps before exit
delete bmp0;
delete bmp1;
}
//---------------------------------------------------------------------------
void __fastcall TMain::tim_updateTimer(TObject *Sender)
{
// skip if App not yet initialized
if (bmp0==NULL) return;
if (bmp1==NULL) return;
int x,y,i,a;
union _color
{
BYTE db[4];
DWORD dd;
};
// copy bmp0 into bmp1 with light reduction and noise
for (y=0;y<bmp0->Height;y++)
{
_color *p0=(_color*)bmp0->ScanLine[y];
_color *p1=(_color*)bmp1->ScanLine[y];
for (x=0;x<bmp0->Width;x++)
{
p1[x]=p0[x];
for (a=40,i=0;i<10;i++) a+=Random(10); // "gauss" PRNG in range 40 .. 140
for (i=0;i<3;i++) p1[x].db[i]=(DWORD(p1[x].db[i])*a)>>8; // multiply RGB by a/256
}
}
// render frame on App canvas
Canvas->Draw(0,0,bmp1);
// bmp1->SaveToFile("out.bmp");
}
//---------------------------------------------------------------------------
input image:
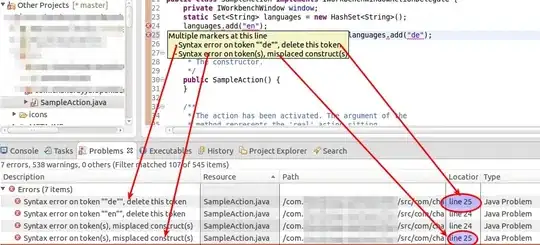
output image:
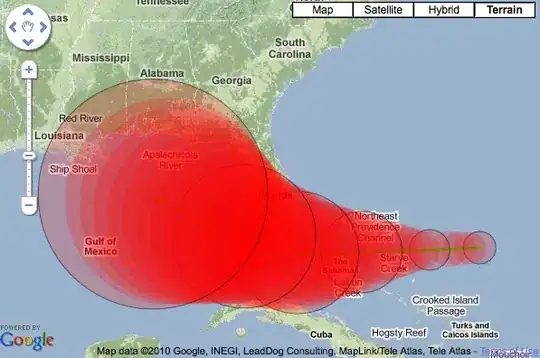
You can play with the PRNG properties to tweak light and noissyness.