To well answer this question, we first need to get ourselves familiarized with two concepts: row-major implementation and column-major implementation.
In Row-Major Implementation, the elements of a row of an array are placed next to those of the previous row elements. All row elements are placed in contiguous memory addresses. Whereas in Column-Major Implementation, the column-wise elements are placed next to each other in contiguous memory allocations.
For more explanation: https://en.wikipedia.org/wiki/Row-_and_column-major_order
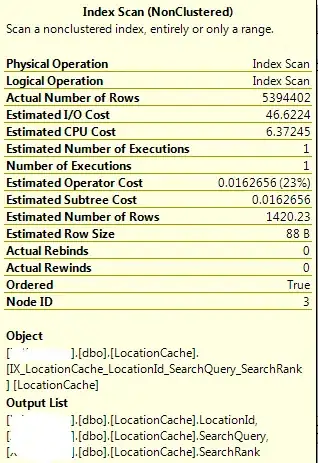
Now coming back, C/C++ language is based on Row-Major implementation. Thus, even if we don't give the number of rows while declaring an array, the number of columns provided during the declaration would be sufficient for the compiler to decide the row to which an element should belong to.
For example, in the following way of declaration and initialization:
int a[][3] = {1,2,3,4,5,6}
Though the number of rows is not mentioned, the number of columns provided would help the compiler decide that the elements 1,2,3
should belong to the first row of the matrix, and 4,5,6
elements should belong to the second row, since each row should only contain 3
columns. So this type of declaration and initialization is valid.
On the other hand, in the following example,
int a[2][] = {1,2,3,4,5,6}
It is only given that there should be 2 rows. But since C a is row-major implementation-based language, though it knows that there should be 2 rows, it doesn't know the offset of the number of columns each row should have. So it doesn't have an answer for the question: "from which element should the second row start?".
Since the second row will be filled only after filling the first row in row-major implementation, and here since the number of columns is unknown, the compiler will never be able to get the answer for the above question, and thus it doesn't know which elements should do go the next row. It doesn't know the number of elements in each row due to the lack of offset on the number of columns each row should have.
And hence, regardless of the number of rows being mentioned or not, it is mandatory to mention the number of columns during array declaration in C.