Since I couldn't download the kaggle data presented, I got the AAPL stock price from Yahoo Finance and used it as data. For the graph itself, I used the code in kaggle to change the color of each line to make a graph. For the text annotations, I used add_annotation(). The annotated data was set appropriately.
import pandas as pd
from datetime import datetime
import plotly.graph_objects as go
import yfinance as yf
df = yf.download("AAPL", start="2021-06-21", interval='1m', end="2021-06-22")
df.reset_index(inplace=True)
df.columns =['time', 'open', 'high', 'low', 'close', 'adj close', 'volume']
# Create a working copy of the last 2 hours of the data
btc_data = df.iloc[-240:].copy()
# Define the parameters for the Bollinger Band calculation
ma_size = 20
bol_size = 2
# Convert the timestamp data to a human readable format
btc_data.index = pd.to_datetime(btc_data.time, unit='ms')
# Calculate the SMA
btc_data.insert(0, 'moving_average', btc_data['close'].rolling(ma_size).mean())
# Calculate the upper and lower Bollinger Bands
btc_data.insert(0, 'bol_upper', btc_data['moving_average'] + btc_data['close'].rolling(ma_size).std() * bol_size)
btc_data.insert(0, 'bol_lower', btc_data['moving_average'] - btc_data['close'].rolling(ma_size).std() * bol_size)
# Remove the NaNs -> consequence of using a non-centered moving average
btc_data.dropna(inplace=True)
# Create an interactive candlestick plot with Plotly
fig = go.Figure(data=[go.Candlestick(x = btc_data.index,
open = btc_data['open'],
high = btc_data['high'],
low = btc_data['low'],
showlegend = False,
close = btc_data['close'])])
# Plot the three lines of the Bollinger Bands indicator
parameter = ['moving_average', 'bol_lower', 'bol_upper']
colors = ['blue', 'orange', 'orange']
for param,c in zip(parameter, colors):
fig.add_trace(go.Scatter(
x = btc_data.index,
y = btc_data[param],
showlegend = False,
line_color = c,
mode='lines',
line={'dash': 'solid'},
marker_line_width=2,
marker_size=10,
opacity = 0.8))
# Add title and format axes
fig.update_layout(
title='Bitcoin price in the last 2 hours with Bollinger Bands',
yaxis_title='BTC/USD')
x = '2021-06-21 10:56:00'
y = df['close'].max()
fig.add_annotation(x=x, y=y,
text='\U000025bc',
showarrow=False,
yshift=10)
xx = '2021-06-21 09:50:00'
yy = df['close'].min()
fig.add_annotation(x=xx, y=yy,
text='\U000025b2',
showarrow=False,
yshift=10)
fig.show()
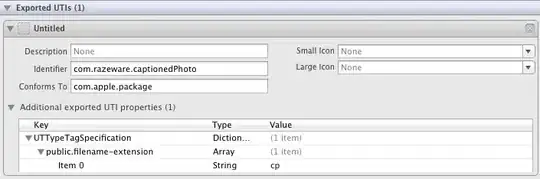