In order to copy a cell without taking the formatting you could also just write the value of your desired cell. Excel will then just take the "Value" within a cell and write it to a different one without including the formatting.
Something like this:
Sub Test1()
Dim Ws As Worksheet
Set Ws = ActiveSheet
Ws.Cells(7, 2).Value = Ws.Cells(6, 2).Value
End Sub
Here i refer to the cells themselves instead of the range, but it can also be done using a range.
Sub Test1()
Dim Ws As Worksheet
Set Ws = ActiveSheet
Ws.Range("B7").Value = Ws.Range("B6").Value
End Sub
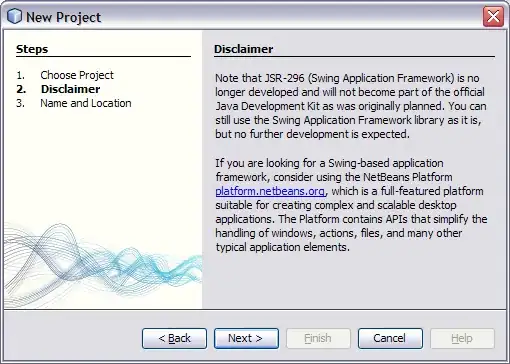
After filling the cell with just the value you can change the formatting however you like by using a With statement.
Sub Test1()
Dim Ws As Worksheet
Set Ws = ActiveSheet
Ws.Range("B7").Value = Ws.Range("B6").Value
With Ws.Range("B7")
.Font.Name = "Arial" 'Name of the font you want to use
.Font.Color = vbYellow 'Color of the font you want to use
.Font.Size = 22 'Size of your font
.Interior.Color = vbRed 'Color of the cell
.Font.Bold = True 'Making the text bold True/False
End With
End Sub