As mentioned in the comments JEditorPane is quite limited (mainly doesn't support JS which is required).
For example:
import javax.swing.JEditorPane;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
void setup(){
showWebPage();
println("done");
}
void draw(){
}
void showWebPage(){
try{
JEditorPane website = new JEditorPane("https://www.google.nl/maps/@51.7385025,-2.6407162,3a,79y,132.82h,79.64t/data=!3m6!1e1!3m4!1sMe0y36wXo7_CSHvur_4kPg!2e0!7i13312!8i6656?hl=nl");
website.setEditable(false);
JFrame frame = new JFrame("Google");
frame.add(new JScrollPane(website));
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(800, 600);
frame.setVisible(true);
}catch(IOException e){
e.printStackTrace();
}
}
outputs:
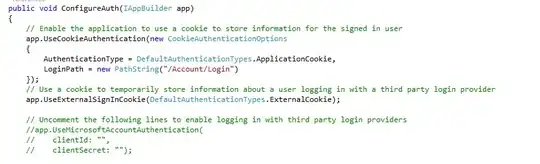
If you don't need interactivity you might be able to get away with the Google Street View Static API
Here's one of the examples:
PImage streetView;
void setup() {
size(400, 400);
streetView = loadImage("https://maps.googleapis.com/maps/api/streetview?size=400x400&location=47.5763831,-122.4211769&fov=80&heading=70&pitch=0&key=AIzaSyA3kg7YWugGl1lTXmAmaBGPNhDW9pEh5bo&signature=hg7yTczCuAp4fwIWFySlSr_vq7o=&filename=streetview.png");
}
void draw() {
image(streetView, 0, 0);
}
There are a couple of caveats:
- As the Street View API documentation mentions, you need to get an API Key and Signature
loadImage()
needs an extension to know how to decode data, hence the &filename=streetview.png
I added at the end (could be something like &ext=.png
for example, as long as it's a valid URL and ends with the extension for Processing)
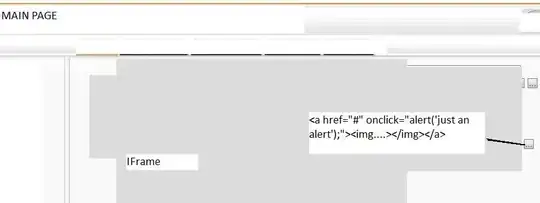
If you need to load a dynamic webpage you can try your luck with JavaFX. I'm not an experienced with it, but hopefully you can get something off the ground with the FX2D
renderer in Processing
Personally I find using WebAPIs in Java a bit cumbersome/verbose.
Depending on how you plan to integrate the street view in a larger application you can consider using the Street View Service directly in JavaScript and the Processing functionality with p5.js. If you need native system functionality you could load the p5.js front-end in an electron app