You can do this with prompt_toolkit
here is the documentation if you need:
You can add Syntax Highlighting to input by the following as given in examples:
Adding syntax highlighting is as simple as adding a lexer. All of the Pygments lexers can be used after wrapping them in a PygmentsLexer. It is also possible to create a custom lexer by implementing the Lexer abstract base class.
from pygments.lexers.html import HtmlLexer
from prompt_toolkit.shortcuts import prompt
from prompt_toolkit.lexers import PygmentsLexer
text = prompt('Enter HTML: ', lexer=PygmentsLexer(HtmlLexer))
print('You said: %s' % text)
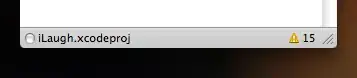
In the same way as above you can create custom prompt_toolkit.lexers.Lexer
for calculator highlighting just like the following example. Here I create a custom helper class:
from typing import Callable
from prompt_toolkit.document import Document
from prompt_toolkit.formatted_text.base import StyleAndTextTuples
from prompt_toolkit.formatted_text import FormattedText
from prompt_toolkit.shortcuts import prompt
import prompt_toolkit.lexers
import re
class CustomRegexLexer(prompt_toolkit.lexers.Lexer):
def __init__(self, regex_mapping):
super().__init__()
self.regex_mapping = regex_mapping
def lex_document(self, document: Document) -> Callable[[int], StyleAndTextTuples]:
def lex(_: int):
line = document.text
tokens = []
while len(line) != 0:
for pattern, style_string in self.regex_mapping.items():
match: re.Match = pattern.search(line)
if not match:
continue
else:
# print(f"found_match: {match}")
pass
match_string = line[:match.span()[1]]
line = line[match.span()[1]:]
tokens.append((style_string, match_string))
break
return tokens
return lex
Now with the above helper class implemented we can create our regex patterns and their respective styles, to learn more about what you can have in styling string go to this page
# Making regex for different operators. Make sure you add `^` anchor
# to the start of all the patterns
operators_allowed = ["+", "-", "/", "*", "(", ")", "=", "^"]
operators = re.compile("^["+''.join([f"\\{x}" for x in operators_allowed])+"]")
numbers = re.compile(r"^\d+(\.\d+)?")
text = re.compile(r"^.")
regex_mapping = {
operators: "#ff70e5", # Change colors according to your requirement
numbers: "#ffa500",
text: "#2ef5ff",
}
MyCalculatorLexer = CustomRegexLexer(regex_mapping)
With the lexers created you can now use the lexer in the function prompt:
text = prompt("Enter Equation: ", lexer=MyCalculatorLexer)
# Or
def input_maths(message):
return prompt(message, lexer=MyCalculatorLexer)
text = input_maths("Enter Equation: ")
Here is some example output:
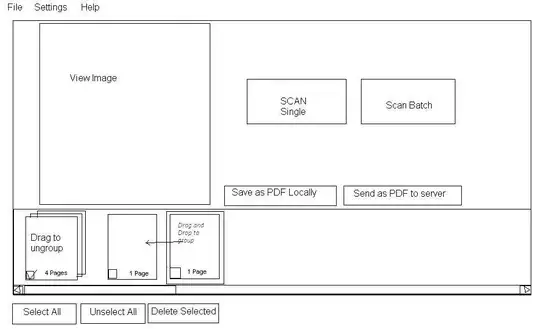
And now everything works. Also do check out prompt_toolkit
, you can
create tons of custom things as shown in there gallery