A line break only makes sense if the response is an HTML response (i.e. an HTML page). And a \n
does not render properly as new lines or line breaks, you have to use <br>
or an HTML template + some CSS styling to preserve line breaks.
FastAPI returns, by default, a JSONResponse
type.
Takes some data and returns an application/json
encoded response.
This is the default response used in FastAPI, as you read above.
But you can tell it to use an HTMLResponse
type using the response_class
parameter:
from fastapi import FastAPI
from fastapi.responses import HTMLResponse
app = FastAPI()
@app.get('/status', response_class=HTMLResponse)
def get_func():
output = 'this output should <br> have a line break'
return output
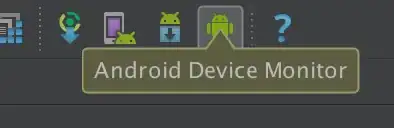
Or, for better control, use an actual HTML template. FastAPI supports Jinja2 templates, see section on FastAPI Templates.
proj/templates/output.html
<html>
<head>
</head>
<body>
<p>This output should have a <br>line break.</p>
<p>Other stuff: {{ stuff }}</p>
</body>
</html>
proj/main.py
from fastapi import FastAPI, Request
from fastapi.responses import HTMLResponse
from fastapi.templating import Jinja2Templates
app = FastAPI()
templates = Jinja2Templates(directory="templates")
@app.get('/status', response_class=HTMLResponse)
def get_func(request: Request):
return templates.TemplateResponse("output.html", {"request": request, "stuff": 123})
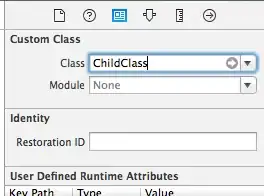
With HTML templates, you can then use CSS styling to preserve line breaks.