Does this solve your problem ... simply join on ID and CLASS with predicates sorting out the dates?
select * ,
datediff(days,greatest(TABLE_1.START_DATE,TABLE_2.START_DATE )
,least( TABLE_1.END_DATE,TABLE_2.END_DATE )) overlapping_days
from TABLE_1 inner join TABLE_2
on TABLE_1.id = TABLE_2.id AND TABLE_1.CLASS <> TABLE_2.CLASS
where TABLE_1.START_DATE between TABLE_2.START_DATE and TABLE_2.END_DATE
or TABLE_1.END_DATE between TABLE_2.START_DATE and TABLE_2.END_DATE
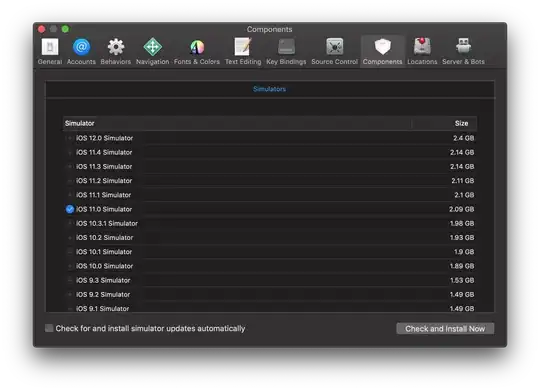
Or am I missing something? (code to copy/paste into snowflake)
with TABLE_1 as (
select 1 ID, TO_DATE('10-FEB-21','DD-MON-YY') START_DATE, TO_DATE('17-FEB-21','DD-MON-YY') END_DATE, 'A' CLASS
union select 1 ID, TO_DATE('15-FEB-21','DD-MON-YY') START_DATE, TO_DATE('21-FEB-21','DD-MON-YY') END_DATE, 'A' CLASS
union select 1 ID, TO_DATE('11-MAR-21','DD-MON-YY') START_DATE, TO_DATE('21-MAR-21','DD-MON-YY') END_DATE, 'A' CLASS
union select 1 ID, TO_DATE('19-APR-21','DD-MON-YY') START_DATE, TO_DATE('14-MAY-21','DD-MON-YY') END_DATE, 'A' CLASS
union select 1 ID, TO_DATE('10-MAY-21','DD-MON-YY') START_DATE, TO_DATE('11-JUN-21','DD-MON-YY') END_DATE, 'A' CLASS)
, TABLE_2 AS (
SELECT 1 ID, TO_DATE('16-JAN-21','DD-MON-YY') START_DATE, TO_DATE('28-FEB-21','DD-MON-YY') END_DATE, 'B' CLASS
UNION SELECT 1 ID, TO_DATE('15-MAR-21','DD-MON-YY') START_DATE, TO_DATE('14-APR-21','DD-MON-YY') END_DATE, 'B' CLASS
UNION SELECT 1 ID, TO_DATE('12-APR-21','DD-MON-YY') START_DATE, TO_DATE('12-MAY-21','DD-MON-YY') END_DATE, 'B' CLASS
UNION SELECT 1 ID, TO_DATE('09-MAY-21','DD-MON-YY') START_DATE, TO_DATE('14-MAY-21','DD-MON-YY') END_DATE, 'B' CLASS
UNION SELECT 1 ID, TO_DATE('07-JUN-21','DD-MON-YY') START_DATE, TO_DATE('01-JUL-21','DD-MON-YY') END_DATE, 'B' CLASS)
select * , datediff(days, greatest(TABLE_1.START_DATE,TABLE_2.START_DATE ) , least( TABLE_1.END_DATE,TABLE_2.END_DATE )) overlapping_days from TABLE_1 inner join TABLE_2
on TABLE_1.id = TABLE_2.id AND TABLE_1.CLASS <> TABLE_2.CLASS
where TABLE_1.START_DATE between TABLE_2.START_DATE and TABLE_2.END_DATE
or TABLE_1.END_DATE between TABLE_2.START_DATE and TABLE_2.END_DATE