To shade a contour area, you can use plt.contourf()
. You need to provide at least two levels to be colored in between, e.g. using a very small value together with zero. The function accepts an explicit list of colors (or can work with a colormap).
ax.spines['left'].set_position('center')
can set the y-axis at the zero of x, instead of the default placement at the border. ax.set_ylabel('y', loc='top')
sets the y-label at the top (default is in the center).
import matplotlib.pyplot as plt
import numpy as np
fig = plt.figure(figsize=(10, 7))
x = np.linspace(-5.0, 5.0, 100)
y = np.linspace(-5.0, 5.0, 100)
X, Y = np.meshgrid(x, y)
F = X ** 2 + Y ** 2 - 1
plt.contourf(X, Y, F, [-100, 0], colors=['dodgerblue'])
ax = plt.gca()
ax.spines['left'].set_position('center')
ax.spines['bottom'].set_position('center')
ax.spines['right'].set_visible(False)
ax.spines['top'].set_visible(False)
ax.set_xlabel('x', loc='right')
ax.set_ylabel('y', loc='top')
plt.show()
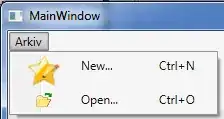
Note that Python's symbolic math package sympy
does something similar without the need to create a grid.
from sympy import plot_implicit
from sympy.abc import X, Y
plot1 = plot_implicit(X ** 2 + Y ** 2 < 1)
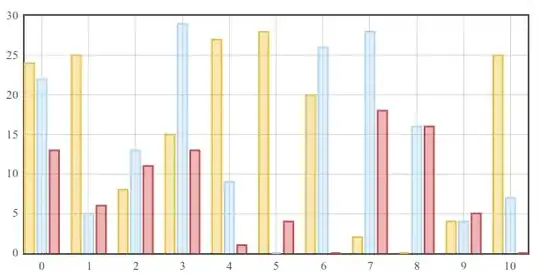
To make changes to the sympy plot, you can either access the ax
via plot1._backend.ax[0]
, or use matplotlib with plt.gca()
and plt.gcf()
as follows:
from sympy import plot_implicit
from sympy.abc import X, Y
plot1 = plot_implicit(X ** 2 + Y ** 2 < 1)
import matplotlib.pyplot as plt
ax = plt.gca()
ax.set_xlabel('new x label', ha='right')
ax.set_ylabel('new y label', ha='right')
ax.set_aspect('equal') # use same transformation for x and y, so circles aren't deformed
fig = plt.gcf()
fig.set_size_inches(8, 8)
fig.savefig('test_circle.png', dpi=100)