Python list comprehensions are for loops executed in a list to generate a new list.The reason python list comprehensions are evaluated backward from or right to left is because usually anything inside a bracket( []
, {}
, ()
) in python is executed from right to left with just a few exeptions .Another thing to note is that a string is an iterable (lists
,tuples
, sets
, dictionaries
, numpy arrays) concatenating characters so it can be iterated over like a list.
List Comprhension form:
new_list = [item for item in my_list]
This will have the same effect:
for item in my_list:
my_list.append(item)
Since a strings is an iterable of characters you can do this:
my_list = [character for character in 'Hello world!']
print(list)
Output:
['h', 'e', 'l', 'l', 'o', ' ', 'w', 'o', 'r', 'l', 'd']
Your list comprehension can also be written as:
my_list = []
for character in 'Hello world':
my_list.append(character)
print(my_list)
I am also pointing out that you shouldn't use built in methods(such as List
) as variable names because when you do you overide them which will bar you from using that method in the future.
Here is a complete list of all builins as of python 3.9.6:
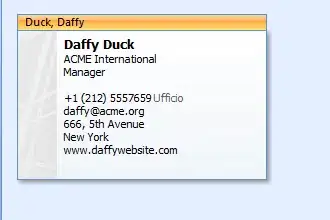