I am not quite sure what your desired output is. For now I'll assume you want to know the center and radius of your volcano.
My approach is to set up a volcano generator and wiggle on it's parameters till it generates basically yours. Then since I know how it was generated I can tell you all about it.
import torch
import torch.nn as nn
import torch.optim
class Net(nn.Module):
def __init__(self):
super().__init__()
self.foci = nn.Parameter(torch.tensor([[30.0,30.0],[30.0,30.0]]))
self.r = nn.Parameter(torch.tensor((20.0)))
self.a = nn.Parameter(torch.tensor((-0.1)))
self.b = nn.Parameter(torch.tensor((0.01)))
def generate_volcano(self):
eps = 1e-15
x,y = torch.meshgrid(torch.arange(60.0), torch.arange(60.0))
d1 = ((self.foci[0,0] - x)**2+(self.foci[0,1] - y)**2 + eps)**(1/2)
d2 = ((self.foci[1,0] - x)**2+(self.foci[1,1] - y)**2 + eps)**(1/2)
return torch.sigmoid(self.a*(d1+d2-self.r)**2+self.b)
n = 10**5
optimizer = optim.Adam(net.parameters())
torch_image = torch.from_numpy(image.astype('float32'))
loss_funcition = nn.MSELoss()
for i in range(n):
optimizer.zero_grad()
volcano = net.generate_volcano()
loss = loss_funcition(volcano, torch_image)
loss.backward()
optimizer.step()
if i % (n//8) == 0:
print('loss', loss.item())
This gives me a "volcano" like this
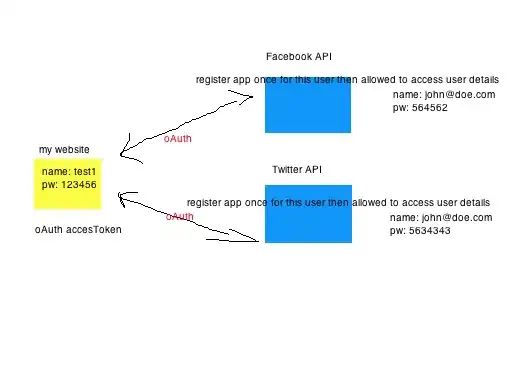
with focal points [32.1, 29.6],[32.1, 29.6]
and radius 38.527
.