Though there are multiple explanations, I will attempt based on my understanding using the IL code of various scenarios.
- Instantiate the list in the constructor
In this case, when the object is constructed by this constructor it would first make a call to instance void [System.Runtime]System.Object::.ctor() and then construct the list
public class ObjectA
{
public List<string> MyList { get; set; }
public ObjectA()
{
this.MyList = new List<string>();
}
}
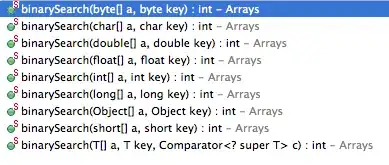
- Instantiate the list at the declaration
In this case, when the object is constructed it would first construct the list and then make a call to the default constructor instance void [System.Runtime]System.Object::.ctor() and then initialize the list
public class ObjectA
{
public List<string> MyList { get; set; } = new List<string>();
}
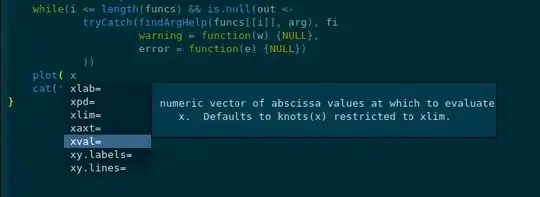
- Instantiate the list at the declaration but the class has one constructor
In this case, compiler has injected the code for the list instantiation in the constructor
public class ObjectA
{
public List<string> MyList { get; set; } = new List<string>();
public ObjectA()
{
}
}
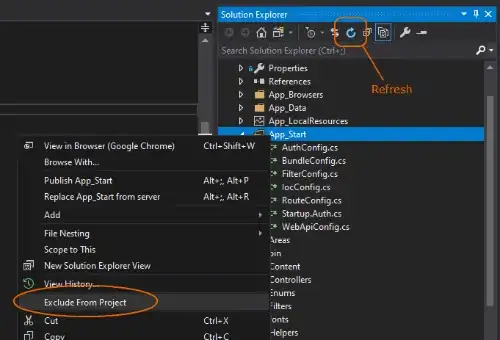
- Instantiate the list at the declaration, and create the class with different constructor
In this case we can see the compiler has injected the code for the list instantiation in the new constructor too
public class ObjectA
{
public List<string> MyList { get; set; } = new List<string>();
public ObjectA(int x)
{
}
}
public class ObjectB:ObjectA
{
public ObjectB(int x):base(x)
{
}
}
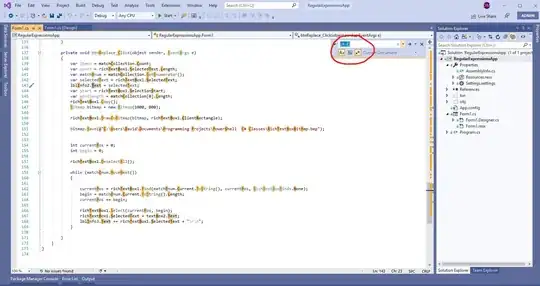
- Instantiate the list at the declaration, calss has two constructors and a derived class
In this case, the compiler injected the list instantiation code in both the constructors. This would not happen in the case where we
instantiate the list in the constructor
public class ObjectA
{
public List<string> MyList { get; set; } = new List<string>();
public ObjectA()
{
}
public ObjectA(int x)
{
}
}
public class ObjectB:ObjectA
{
public ObjectB(int x):base(x)
{
}
}
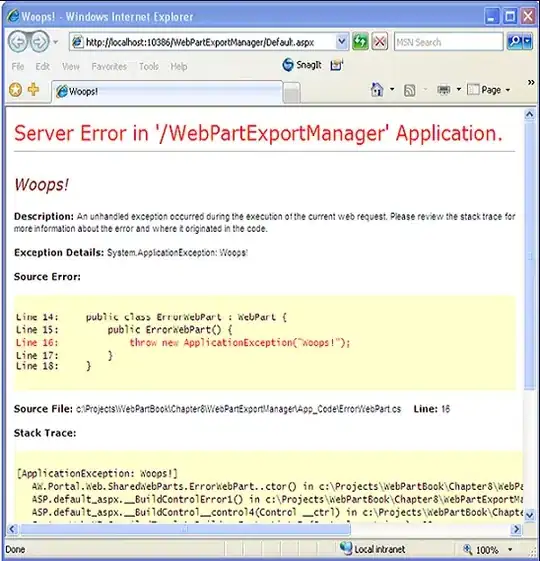
To summarise, In case of lists or similar objects if you have multiple constructors. It's developer's responsibility to ensure all required objects are initialized before the use. The complexity increases when this class gets derived. If we follow the clean code practices, smaller classes I would go with initiaize at declaration. But if the field objects are going to be big, doing some work at their construction, I would initialize them when required (This requires safety)