In general, this problem isn't as simple as you may think. I suppose you are imagining a desktop like this:
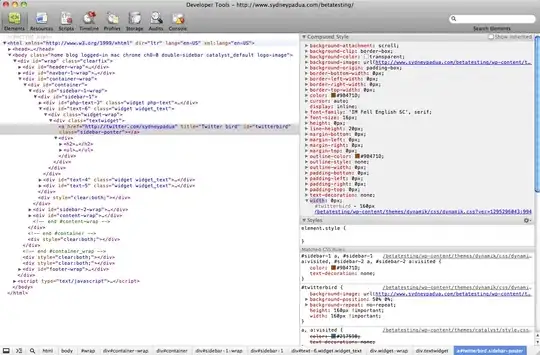
In this case, I assume you want the window to be placed like this:
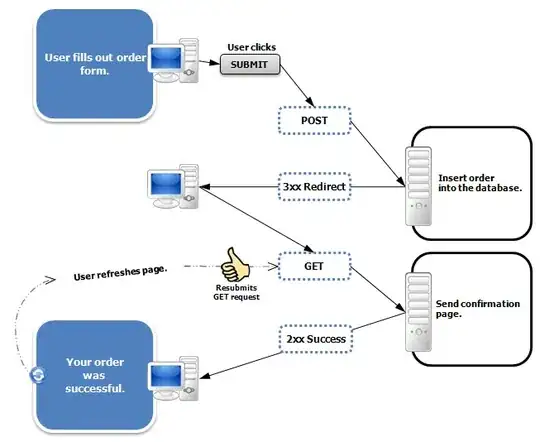
However, what if the user has this layout:

Do you want
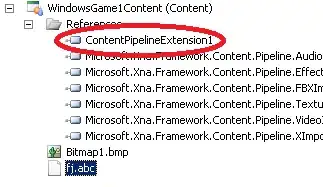
(entire window visible, but some screen space unused) or

(no unused space, but some parts of the window not visible)?
If you want to use the full virtual desktop space -- the last case -- it is easy though:
BoundsRect := Screen.DesktopRect;
This will do the expected thing in a simple setup, and the "no unused space, but some parts of the window might not be visible" thing in general.
Also be aware that Windows doesn't like that windows behave like this, so the user might not get a nice experience using the app.
In general, don't do this.
Please note that even a two-monitor setup, in which both monitors are landscape, can be non-trivial:
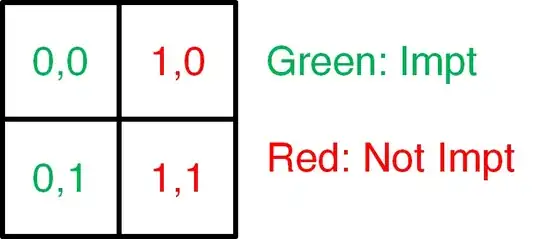
The geometry may be non-trivial even if both monitors are the same size:
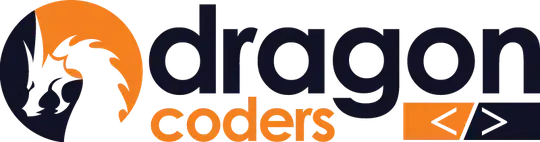