I would do this slightly different.
LOGIC
- Find the last row in column D as shown HERE
- Loop through the range and then check if the cell in
D
has some value. i.e the new worksheet name cannot be blank.
- Loop through the range and then check if the value from the
D
cell can be used to name a sheet. We check for invalid characters and character limit using IsValidSheetName()
- The next check we do is to check if there is already a sheet with that name. For this, I am using
DoesSheetExist()
- Only if the above checks pass, do we add a sheet and rename it. In your current scenario, you will end up with lot of worksheets if there are errors.
CODE
Is this what you are trying? I have commented the code so you should not have a problem understanding it. But if you do, then, simply ask.
Option Explicit
Sub AddSheets()
Dim Hoja As Worksheet
Dim Libro As Workbook
Dim LRow As Long
Dim i As Long
Dim NewSheetName As String
Set Libro = ThisWorkbook
Set Hoja = Libro.Sheets("Hoja 2")
Application.ScreenUpdating = False
With Hoja
'~~> Find last row in column D
LRow = .Range("D" & .Rows.Count).End(xlUp).Row
For i = 1 To LRow
NewSheetName = .Range("D" & i).Value2
'~~> Check if the worksheet name is not blank
If Len(Trim(NewSheetName)) = 0 Then
Debug.Print "The worksheet name cannot be blank"
'~~> Check if the sheet name is valid
ElseIf IsValidSheetName(NewSheetName) = False Then
Debug.Print "The sheet name " & NewSheetName & _
" cannot have length more than 31 " & _
"characters. Neither it can contain the characters /,\,[,],*,?,:"
'~~> Check if there is no other sheet with that name
ElseIf DoesSheetExist(NewSheetName) Then
Debug.Print "There is already a sheet with the name." & NewSheetName
Else
Libro.Sheets.Add after:=Libro.Sheets(Libro.Sheets.Count)
ActiveSheet.Name = NewSheetName
End If
Next i
End With
Application.ScreenUpdating = True
End Sub
'~~> Function to check if the sheet name is valid
Private Function IsValidSheetName(userinput As Variant) As Boolean
Dim IllegalChars As Variant
Dim i As Long
IllegalChars = Array("/", "\", "[", "]", "*", "?", ":")
If Len(userinput) > 31 Then Exit Function
For i = LBound(IllegalChars) To UBound(IllegalChars)
If InStr(userinput, (IllegalChars(i))) > 0 Then Exit Function
Next i
IsValidSheetName = True
End Function
'~~> Function to check if worksheet exists
Private Function DoesSheetExist(userinput As Variant) As Boolean
Dim wsh As Worksheet
On Error Resume Next
Set wsh = ThisWorkbook.Sheets(userinput)
On Error GoTo 0
If Not wsh Is Nothing Then DoesSheetExist = True
End Function
IN ACTION
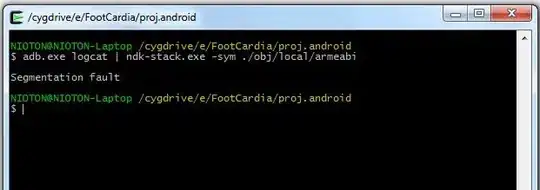