is it possible to change the thumb of a Slider in SwiftUI like you can with a UISlider in UIKit? With a UISlider all you have to do is:
self.setThumbImage(UIImage(named: "Play_black"), for: .normal)
Asked
Active
Viewed 5,732 times
2

aFella
- 145
- 1
- 7
-
Does this answer your question? [How customise Slider blue line in SwiftUI?](https://stackoverflow.com/questions/57101754/how-customise-slider-blue-line-in-swiftui) – excitedmicrobe Aug 27 '21 at 15:19
3 Answers
9
You can do this with SwiftUI-Introspect.
You are basically just accessing the underlying UISlider
from SwiftUI's Slider
, and then setting it there. This is much easier than creating a custom slider or making a representable.
Code:
struct ContentView: View {
@State private var value: Double = 0
var body: some View {
Slider(value: $value)
.introspectSlider { slider in
slider.setThumbImage(UIImage(named: "Play_black"), for: .normal)
}
}
}
Result (temporary image):
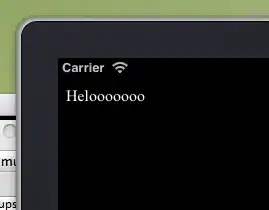

George
- 25,988
- 10
- 79
- 133
5
You can do this using UIKit's appearance
in SwiftUI.
Example:
struct CustomSlider : View {
@State private var value : Double = 0
init() {
let thumbImage = UIImage(systemName: "circle.fill")
UISlider.appearance().setThumbImage(thumbImage, for: .normal)
}
var body: some View {
Slider(value: $value)
}
}
-
Your answer could be improved with additional supporting information. Please [edit] to add further details, such as citations or documentation, so that others can confirm that your answer is correct. You can find more information on how to write good answers [in the help center](/help/how-to-answer). – Community Jul 18 '22 at 14:41
-
This is awesome! A great solution that avoids using a third-party framework or building a custom slider from scratch. Thank you! – Josh Brown Jul 25 '22 at 22:08
1
You can't simply modify the thumb of a Slider in SwiftUI. But, you can create a custom slider though.
Here is an example: https://swiftuirecipes.com/blog/custom-slider-in-swiftui