If you have a dataframe like this one, with date on index and some columns with values:
df = pd.DataFrame({'date': pd.date_range(start = '2021-01-01', end = '2021-01-05', freq = 'H')})
df['value 1'] = np.random.random(len(df))
df['value 2'] = np.random.random(len(df))
df = df.set_index('date')
value 1 value 2
date
2021-01-01 00:00:00 0.374540 0.427541
2021-01-01 01:00:00 0.950714 0.025419
2021-01-01 02:00:00 0.731994 0.107891
2021-01-01 03:00:00 0.598658 0.031429
2021-01-01 04:00:00 0.156019 0.636410
2021-01-01 05:00:00 0.155995 0.314356
2021-01-01 06:00:00 0.058084 0.508571
2021-01-01 07:00:00 0.866176 0.907566
2021-01-01 08:00:00 0.601115 0.249292
2021-01-01 09:00:00 0.708073 0.410383
You can define start and end time of the job and use them to filter your dataframe; where hour is out of this bound, you set data to None
:
start_working_hour = 8
end_working_hour = 17
filt = (df.index.hour < start_working_hour) | (df.index.hour > end_working_hour)
df.loc[filt] = None
Complete Code
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
df = pd.DataFrame({'date': pd.date_range(start = '2021-01-01', end = '2021-01-05', freq = 'H')})
df['value 1'] = np.random.random(len(df))
df['value 2'] = np.random.random(len(df))
df = df.set_index('date')
start_working_hour = 8
end_working_hour = 17
filt = (df.index.hour < start_working_hour) | (df.index.hour > end_working_hour)
df.loc[filt] = None
df.plot()
plt.show()
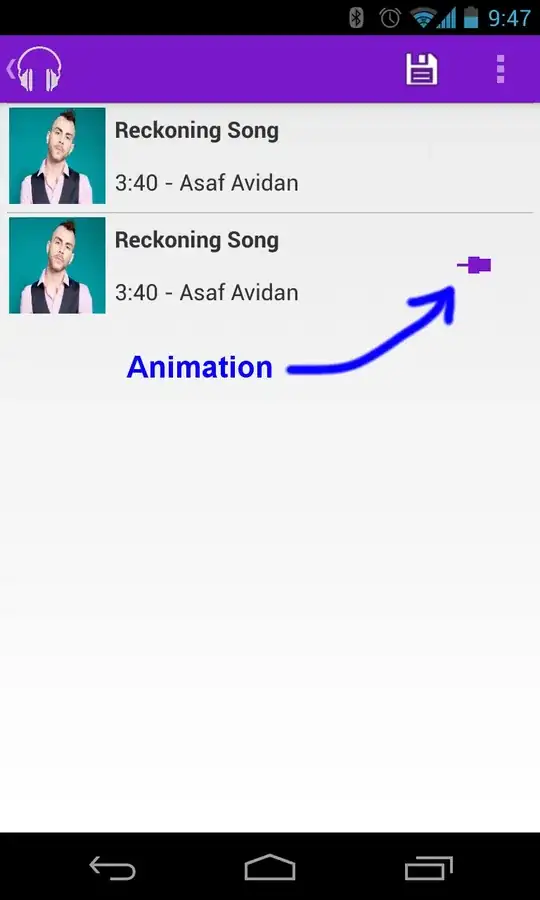
If you want to remove white gaps between lines so as to have continuous lines, taking inspiration from this answer, you should plot using range(df.index.size)
as x axis, then you need to adjust x ticks.
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from datetime import time
df = pd.DataFrame({'date': pd.date_range(start = '2021-01-01', end = '2021-01-05', freq = 'H')})
df['value 1'] = np.random.random(len(df))
df['value 2'] = np.random.random(len(df))
df = df.set_index('date')
start_working_hour = 8
end_working_hour = 16
hour_step = 2
filt = (start_working_hour <= df.index.hour) & (df.index.hour <= end_working_hour)
df = df.loc[filt]
fig, ax = plt.subplots(figsize = (15, 5))
ax.plot(range(df.index.size), df['value 1'], label = 'value 1')
ax.plot(range(df.index.size), df['value 2'], label = 'value 2')
ax.grid(axis='x', alpha=0.3)
ticks_date = df.index.indexer_at_time(time(start_working_hour).strftime('%H:%M'))
ticks_time = np.arange(df.index.size)[df.index.minute == 0][::hour_step]
ax.set_xticks(ticks_date)
ax.set_xticks(ticks_time, minor=True)
labels_date = [maj_tick.strftime('\n%d-%b').replace('\n0', '\n') for maj_tick in df.index[ticks_date]]
labels_time = [min_tick.strftime('%H:%M') for min_tick in df.index[ticks_time]]
ax.set_xticklabels(labels_date)
ax.set_xticklabels(labels_time, minor=True)
ax.figure.autofmt_xdate(rotation=0, ha='center', which='both')
ax.legend(frameon = True)
plt.show()
