I would strongly discourage using a category to override -drawRect
on a UINavBar as this design will break on a soon to be released iOS version.
It is very easy to create custom images and buttons to the navBar title though. Here's some code snippets I pulled directly out of production code for one of my apps. It creates a custom image button in the navBar's title area, but it's just as easy to create an image instead:
- (void) viewDidLoad {
UIButton *titleButton = [self buttonForTitleView];
[titleButton setTitle:newTitle forState:UIControlStateNormal];
[titleButton sizeToFit];
self.navigationBar.topItem.titleView = titleButton;
}
- (UIButton *) buttonForTitleView {
if (!_buttonForTitleView) {
UIImage *buttonImage = [UIImage imageNamed:@"button_collection_name2"];
UIImage *pressedButtonImage = [UIImage imageNamed:@"button_collection_name2_pressed"];
UIButton *button = [UIButton buttonWithType:UIButtonTypeCustom];
[button setBackgroundImage: buttonImage forState : UIControlStateNormal];
[button setBackgroundImage: pressedButtonImage forState : UIControlStateHighlighted];
[button setFrame:CGRectMake(0, 0, buttonImage.size.width, buttonImage.size.height)];
[button setTitleColor:[UIColor colorWithRed:38.0/255.0 green:38.0/255.0 blue:38.0/255.0 alpha:1.0] forState:UIControlStateNormal];
[button setTitleShadowColor:[UIColor colorWithRed:230.0/255.0 green:230.0/255.0 blue:230.0/255.0 alpha:1.0] forState:UIControlStateNormal];
button.titleLabel.adjustsFontSizeToFitWidth = YES;
button.titleLabel.minimumFontSize = 10.0;
button.titleEdgeInsets = UIEdgeInsetsMake(0.0, 10.0, 0.0, 10.0);
button.titleLabel.shadowOffset = (CGSize){ 0, 1 };
[button addTarget:self action:@selector(presentWidgetCollectionSwitchingViewController:) forControlEvents:UIControlEventTouchUpInside];
_buttonForTitleView = [button retain];
}
return _buttonForTitleView;
}
And this is what the button looks like in the app VideoBot:
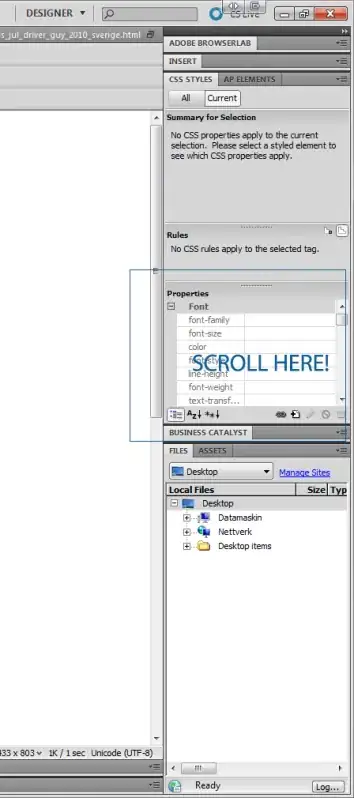
Here's an example of using an image for the navbar title:
UIImage *titleImage = [UIImage imageNamed:@"navbar_title_image"];
UIImageView *titleImageView = [[[UIImageView alloc] initWithImage:titleImage] autorelease];
UIView *container = [[[UIView alloc] initWithFrame:(CGRect){0.0, 0.0, titleImage.size.width, titleImage.size.height}] autorelease];
container.backgroundColor = [UIColor clearColor];
[container addSubview:titleImageView];
// add the view to the title
self.navigationBar.topItem.titleView= container;