If you want if...else
Try this:
import pandas as pd
df = pd.DataFrame({
'Date': ['2020-01-01', '2020-01-02', '2020-01-03', '2020-01-04', '2020-01-05'],
'Value': [1,2,3,4,5]})
df['Value'] = [
0 if (x > '2020-01-02') & (x <= '2020-01-04') else df['Value'].iloc[idx]
for idx, x in enumerate(df['Date'])
]
print(df)
Output:
Date Value
0 2020-01-01 1
1 2020-01-02 2
2 2020-01-03 0
3 2020-01-04 0
4 2020-01-05 5
Or you can use .loc
like below:
import pandas as pd
df = pd.DataFrame({
'Date': ['2020-01-01', '2020-01-02', '2020-01-03', '2020-01-04', '2020-01-05'],
'Value': [1,2,3,4,5]})
df['Date'] = pd.to_datetime(df['Date'])
df.loc[(df['Date'] > '2020-01-02') & (df['Date'] <= '2020-01-04'), 'Value'] = 0
Edit Base On Your Comment (if Date is index):
import pandas as pd
df = pd.DataFrame({
'Date': ['2020-01-01', '2020-01-02', '2020-01-03', '2020-01-04', '2020-01-05'],
'Value': [1,2,3,4,5]})
df.set_index('Date', inplace=True)
df
Output:
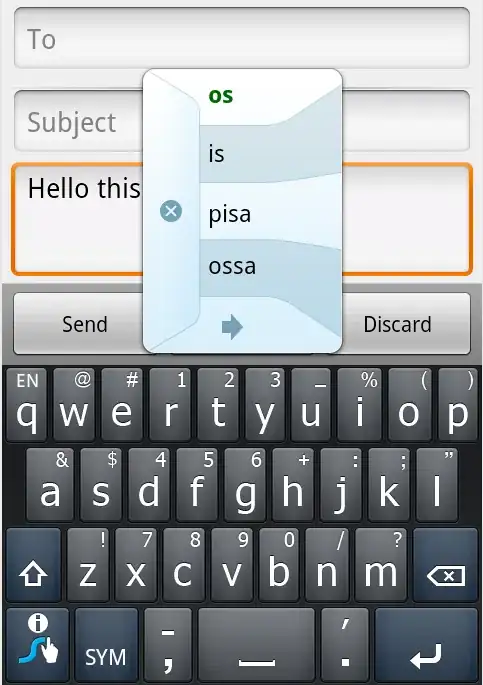
For the above DataFrame you can use df.reset_index()
and like below:
df = df.reset_index()
df['Date'] = pd.to_datetime(df['Date'])
df.loc[(df['Date'] > '2020-01-02') & (df['Date'] <= '2020-01-04'), 'Value'] = 0
With if...else
:
df = df.reset_index()
df['Value'] = [
0 if (x > '2020-01-02') & (x <= '2020-01-04') else df['Value'].iloc[idx]
for idx, x in enumerate(df['Date'])
]