I made an alternative that closely matches your layout using ConstraintLayout
.
Put in your build.gradle
file (module scope inside) inside dependencies
:
// To use constraint layout in compose
implementation "androidx.constraintlayout:constraintlayout-compose:1.0.0-beta02"
NOTE: I'm using icons provided by android studio itself, remember to use yours if they are custom icons. To do this just change the id
parameter of painterResource
referring to the appropriate drawables.
In your compose function that displays the button, use the following code:
Button(
modifier = Modifier
.fillMaxWidth(),
shape = RoundedCornerShape(50),
colors = ButtonDefaults.buttonColors(
backgroundColor = Color(0xFF847EBA),
contentColor = Color.White
),
contentPadding = PaddingValues(horizontal = 4.dp),
onClick = {}
) {
ConstraintLayout(modifier = Modifier.fillMaxWidth()) {
val (startIcon, text, endIcon) = createRefs()
Image(
modifier = Modifier.constrainAs(startIcon) {
start.linkTo(parent.start)
},
painter = painterResource(id = R.drawable.ic_check_circle_outline),
contentDescription = null
)
Text(
modifier = Modifier.constrainAs(text) {
start.linkTo(startIcon.end, margin = 8.dp)
bottom.linkTo(parent.bottom)
top.linkTo(parent.top)
},
text = "John Doe"
)
Image(
modifier = Modifier.constrainAs(endIcon) {
end.linkTo(parent.end)
},
painter = painterResource(id = R.drawable.ic_navigate_next),
contentDescription = null
)
}
}
Visual effect:
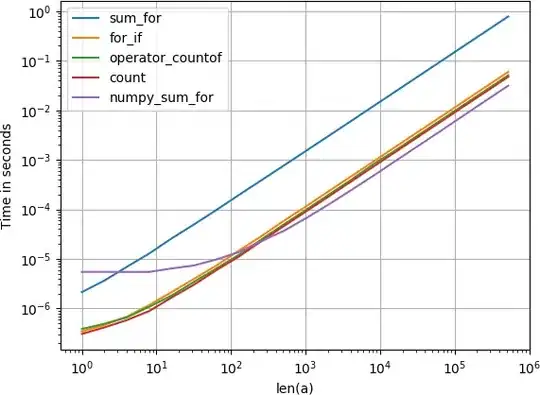