The problem is that your route is expecting 2 types of request body:
That will not work as that breaks not just FastAPI, but general HTTP protocols. FastAPI mentions this in a warning when using File
:
You can declare multiple File
and Form
parameters in a path operation, but you can't also declare Body
fields that you expect to receive as JSON, as the request will have the body encoded using multipart/form-data
instead of application/json
.
This is not a limitation of FastAPI, it's part of the HTTP protocol.
The common solutions, as discussed in Posting a File and Associated Data to a RESTful WebService preferably as JSON, is to either:
- Break the API into 2 POST requests: 1 for the file, 1 for the metadata
- Send it all in 1
multipart/form-data
Fortunately, FastAPI supports solution 2, combining both your Item
model and uploading a file into 1 multipart/form-data
. See the section on Request Forms and Files:
Use File
and Form
together when you need to receive data and files in the same request.
Here's your modified route (I removed db
as that's irrelevant to the problem):
class Item(BaseModel):
name: str
price: float
class PostItem(BaseModel):
name: str
@router.post('/', response_model=PostItem, status_code=status.HTTP_201_CREATED)
def create(
# Here we expect parameters for each field of the model
name: str = Form(...),
price: float = Form(...),
# Here we expect an uploaded file
file: UploadFile = File(...),
):
new_item = Item(name=name, price=price)
print(new_item)
print(file.filename)
return new_item
The Swagger docs present it as 1 form
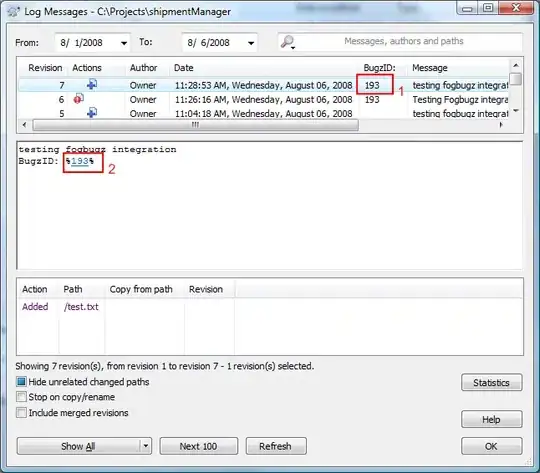
...and you should be able now to send both Item
params and the file in one request.
If you don't like splitting your Item
model into separate parameters (it would indeed be annoying for models with many fields), see this Q&A on fastapi form data with pydantic model.
Here's the modified code where Item
is changed to ItemForm
to support accepting its fields as Form
values instead of JSON:
class ItemForm(BaseModel):
name: str
price: float
@classmethod
def as_form(cls, name: str = Form(...), price: float = Form(...)) -> 'ItemForm':
return cls(name=name, price=price)
class PostItem(BaseModel):
name: str
@router.post('/', response_model=PostItem, status_code=status.HTTP_201_CREATED)
def create(
item: ItemForm = Depends(ItemForm.as_form),
file: UploadFile = File(...),
):
new_item = Item(name=item.name, price=item.price)
print(new_item)
print(file.filename)
return new_item
The Swagger UI should still be the same (all the Item
fields and the file upload all in one form).
For this:
If I leave out the "file: UploadFile = File(...)
" from the function definition, it works correctly
It's not important to focus on this, but it worked because removing File
turned the expected request body back to an application/json
type, so the JSON body would work.
Finally, as a side note, I strongly suggest NOT using request
as a parameter name for your route. Aside from being vague (everything is a request), it could conflict with FastAPI's request: Request
parameter when using the Request object directly.