I'd suggest having a look at Working with Text APIs
A font represents the information necessary to render text
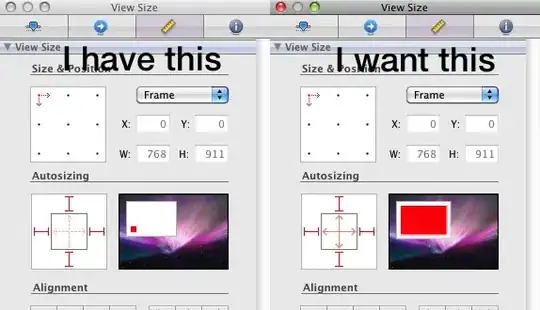
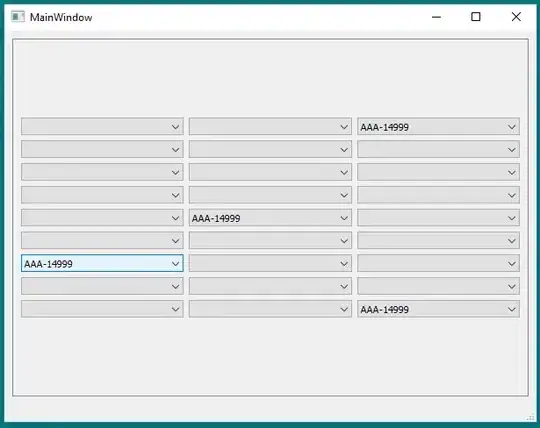
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.util.Arrays;
import java.util.StringJoiner;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
JFrame frame = new JFrame();
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
public TestPane() {
}
@Override
public Dimension getPreferredSize() {
return new Dimension(500, 200);
}
private String[] texts = new String[]{
"oaoaoa g oaoaoa",
"WWWWWW W WWWWWW",
"Prryrr P Prryrr"
};
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
g2d.setColor(Color.RED);
g2d.drawLine(getWidth() / 2, 0, getWidth() / 2, getHeight());
g2d.drawLine(0, getHeight() / 2, getWidth(), getHeight() / 2);
g2d.setColor(Color.BLACK);
FontMetrics fm = g2d.getFontMetrics();
int width = 0;
for (String text : texts) {
width += fm.stringWidth(text);
}
int xPos = (getWidth() - width) / 2;
int yPos = ((getHeight() - fm.getHeight()) / 2) + fm.getAscent();
int offset = 0;
for (String text : texts) {
g2d.drawString(text, xPos + offset, yPos);
offset += fm.stringWidth(text);
}
g2d.dispose();
}
}
}
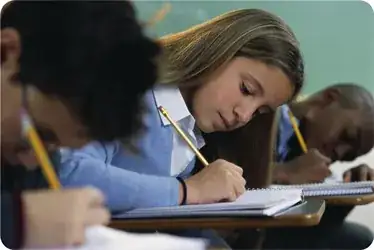
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.util.Arrays;
import java.util.StringJoiner;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
JFrame frame = new JFrame();
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
public TestPane() {
}
@Override
public Dimension getPreferredSize() {
return new Dimension(500, 200);
}
private String[] texts = new String[]{
"oaoaoa g oaoaoa",
"WWWWWW W WWWWWW",
"Prryrr P Prryrr"
};
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
g2d.setColor(Color.RED);
g2d.drawLine(getWidth() / 2, 0, getWidth() / 2, getHeight());
g2d.drawLine(0, getHeight() / 2, getWidth(), getHeight() / 2);
g2d.setColor(Color.BLACK);
FontMetrics fm = g2d.getFontMetrics();
int width = 0;
for (String text : texts) {
width += fm.stringWidth(text);
}
int xPos = (getWidth() - width) / 2;
int yPos = ((getHeight() - fm.getHeight()) / 2) + fm.getAscent();
int offset = 0;
for (String text : texts) {
g2d.drawString(text, xPos + offset, yPos);
offset += fm.stringWidth(text);
}
g2d.dispose();
}
}
}
So, not seeing an issue, but since I have no context to your issue, I'm just guessing
Expanded Example using TextLayout
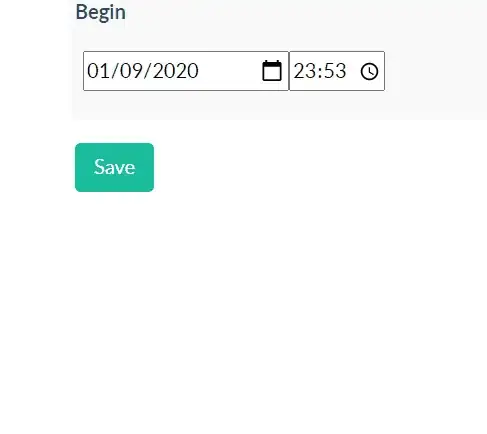
import java.awt.Color;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Rectangle;
import java.awt.RenderingHints;
import java.awt.Shape;
import java.awt.font.FontRenderContext;
import java.awt.font.GlyphVector;
import java.awt.font.TextLayout;
import java.util.HashMap;
import java.util.Map;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
JFrame frame = new JFrame();
frame.add(new TestPane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class TestPane extends JPanel {
public TestPane() {
}
@Override
public Dimension getPreferredSize() {
return new Dimension(500, 200);
}
private String[] texts = new String[]{
"oaoaoa g oaoaoa",
"WWWWWW W WWWWWW",
"Prryrr P Prryrr"
};
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
Graphics2D g2d = (Graphics2D) g.create();
g2d.setRenderingHint(RenderingHints.KEY_ALPHA_INTERPOLATION, RenderingHints.VALUE_ALPHA_INTERPOLATION_QUALITY);
g2d.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);
g2d.setRenderingHint(RenderingHints.KEY_COLOR_RENDERING, RenderingHints.VALUE_COLOR_RENDER_QUALITY);
g2d.setRenderingHint(RenderingHints.KEY_DITHERING, RenderingHints.VALUE_DITHER_ENABLE);
g2d.setRenderingHint(RenderingHints.KEY_FRACTIONALMETRICS, RenderingHints.VALUE_FRACTIONALMETRICS_ON);
g2d.setRenderingHint(RenderingHints.KEY_INTERPOLATION, RenderingHints.VALUE_INTERPOLATION_BILINEAR);
g2d.setRenderingHint(RenderingHints.KEY_RENDERING, RenderingHints.VALUE_RENDER_QUALITY);
g2d.setRenderingHint(RenderingHints.KEY_STROKE_CONTROL, RenderingHints.VALUE_STROKE_PURE);
g2d.setColor(Color.RED);
g2d.drawLine(getWidth() / 2, 0, getWidth() / 2, getHeight());
g2d.drawLine(0, getHeight() / 2, getWidth(), getHeight() / 2);
g2d.setColor(Color.BLACK);
Font font = g2d.getFont();
FontRenderContext frc = g2d.getFontRenderContext();
Map<String, GlyphVector> vectors = new HashMap<>();
double width = 0;
for (String text : texts) {
GlyphVector gv = font.createGlyphVector(frc, text);
vectors.put(text, gv);
width += gv.getVisualBounds().getWidth();
}
int xPos = (int) ((getWidth() - width) / 2.0);
for (String text : texts) {
xPos = render(g2d, text, xPos);
}
g2d.dispose();
}
protected int render(Graphics2D g2d, String text, int xOffset) {
Font font = g2d.getFont();
FontRenderContext frc = g2d.getFontRenderContext();
int xPos = xOffset;
for (char character : text.toCharArray()) {
String value = Character.toString(character);
// Create an layout of the text
TextLayout tl = new TextLayout(value, font, frc);
// Generate a shape of the layout
Shape shape = tl.getOutline(null);
// Align the shape to the center
Rectangle rect = shape.getBounds();
Graphics2D gCopy = (Graphics2D) g2d.create();
int yPos = (getHeight() / 2) + (rect.height / 2);
gCopy.translate(xPos, yPos);
gCopy.fill(shape);
gCopy.dispose();
xPos += (int) (rect.getWidth());
}
return xPos;
}
}
}