Here you can change the scale, offset, and density of the points:
from svg.path import parse_path
from xml.dom import minidom
def get_point_at(path, distance, scale, offset):
pos = path.point(distance)
pos += offset
pos *= scale
return pos.real, pos.imag
def points_from_path(path, density, scale, offset):
step = int(path.length() * density)
last_step = step - 1
if last_step == 0:
yield get_point_at(path, 0, scale, offset)
return
for distance in range(step):
yield get_point_at(
path, distance / last_step, scale, offset)
def points_from_doc(doc, density=5, scale=1, offset=0):
offset = offset[0] + offset[1] * 1j
points = []
for element in doc.getElementsByTagName("path"):
for path in parse_path(element.getAttribute("d")):
points.extend(points_from_path(
path, density, scale, offset))
return points
string = """<svg viewBox="0 0 100 100" xmlns="http://www.w3.org/2000/svg">
<path fill="none" stroke="red"
d="M 10,30
A 20,20 0,0,1 50,30
A 20,20 0,0,1 90,30
Q 90,60 50,90
Q 10,60 10,30 z" />
</svg>"""
doc = minidom.parseString(string)
points = points_from_doc(doc, density=1, scale=5, offset=(0, 5))
doc.unlink()
And you can also visualize those points:
import pygame
from svg.path import parse_path
from xml.dom import minidom
... # other functions and string
def main():
screen = pygame.display.set_mode([500, 500])
screen.fill((255, 255, 255))
doc = minidom.parseString(string)
points = points_from_doc(doc, 0.05, 5, (0, 5))
doc.unlink()
for point in points:
pygame.draw.circle(screen, (0, 0, 255), point, 1)
pygame.display.flip()
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
return
pygame.init()
main()
pygame.quit()
density == 0.05
:
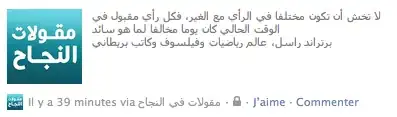
density == 0.1
:
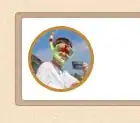
density == 0.5
:
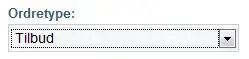
density == 1
:
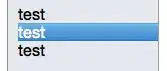
density == 5
:
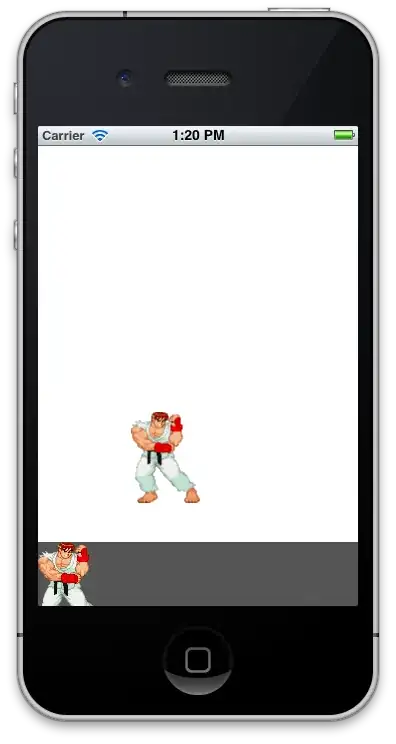