Is there any way to make a scrollbar move using a button in JavaScript/jQuery? I have taken the screenshot from google Sheets; they have that option, but I don't how I can do the same.
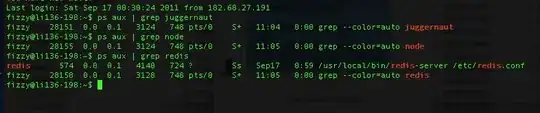
Same image, but close up:
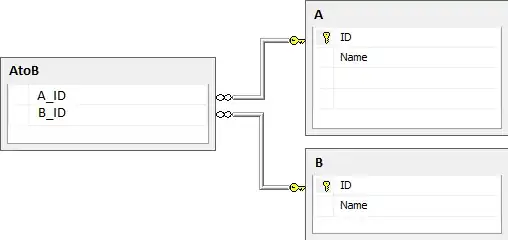
Is there any way to make a scrollbar move using a button in JavaScript/jQuery? I have taken the screenshot from google Sheets; they have that option, but I don't how I can do the same.
Same image, but close up:
They are probably doing something like this:
First, you'll need to hide the default scrollbars, as per this question.
Next, you'll need to implement your own scrollbars, for example something like this project on GitHub.
Finally, when implementing your scrollbars, create buttons, (perhaps using a <button>
tag). In the button click handler, change .scrollTop
or .scrollLeft
to scroll the element incrementally.
For example:
document.getElementById( "my-scroll-left-button" ).onclick = () => {
document.getElementById( "my-scroll-container" ).scrollLeft += 30;
}
document.getElementById( "my-scroll-right-button" ).onclick = () => {
document.getElementById( "my-scroll-container" ).scrollLeft -= 30;
}
document.getElementById( "my-scroll-up-button" ).onclick = () => {
document.getElementById( "my-scroll-container" ).scrollTop -= 30;
}
document.getElementById( "my-scroll-down-button" ).onclick = () => {
document.getElementById( "my-scroll-container" ).scrollTop += 30;
}
Be warned: If you are using CSS scroll-snapping, manually changing .scrollTop
and .scrollLeft
might cause issues in some browsers. (I wouldn't worry about it though, since scroll-snapping is not usually a critical feature.)